C Exercises: Display the pattern like right angle using an asterisk
C For Loop: Exercise-9 with Solution
Write a program in C to display a pattern like a right angle triangle using an asterisk.
* ** *** ****
This C program generates a right-angle triangle pattern using asterisks (*). The user specifies the number of rows for the triangle, and the program uses nested loops to print the pattern. Each row contains an increasing number of asterisks, starting with one asterisk in the first row and adding one more in each subsequent row, creating a right-angle triangle shape.
Visual Presentation:
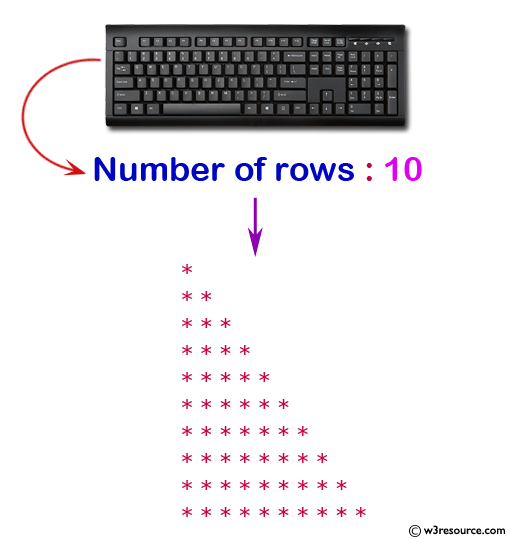
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main() {
int i, j, rows; // Declare variables 'i' and 'j' for loop counters, 'rows' for user input.
printf("Input number of rows : "); // Print a message to prompt user input.
scanf("%d", &rows); // Read the value of 'rows' from the user.
for (i = 1; i <= rows; i++) { // Start a loop to generate rows of asterisks.
for (j = 1; j <= i; j++) // Nested loop to print asterisks based on the current row.
printf("*"); // Print an asterisk.
printf("\n"); // Move to the next line for the next row.
}
}
Output:
Input number of rows : 10 * ** *** **** ***** ****** ******* ******** ********* **********
Explanation:
for (i = 1; i <= rows; i++) { for (j = 1; j <= i; j++) printf("*"); printf("\n"); }
In the above for loop, the variable i is initialized to 1, and the loop will continue as long as i is less than or equal to the value of variable 'rows'. In each iteration of the outer loop, another loop is started with variable j, initialized to 1, and it will continue as long as j is less than or equal to the value of i.
In each iteration of the inner loop, the printf() function will print an asterisk symbol (*) to the console. This symbol will be printed i times, as the value of i is increasing by 1 in each iteration of the outer loop.
After the inner loop completes, the outer loop will print a newline character (\n) to create a new line.
Finally, the outer loop will increment the value of i by 1, and the process will repeat until the condition i<=rows is no longer true.
Flowchart:
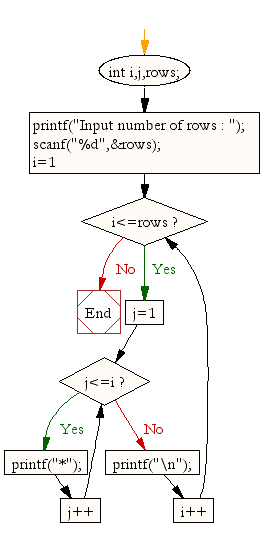
C Programming Code Editor:
Previous: Write a program in C to display the n terms of odd natural number and their sum like:
1 3 5 7 ... n
Next: Write a program in C to display the pattern like right angle triangle with a number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/for-loop/c-for-loop-exercises-9.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics