C Exercises: Display the pattern like right angle triangle using a number
C For Loop: Exercise-10 with Solution
Write a C program to display a pattern like a right angle triangle with a number.
1 12 123 1234
This C program generates a right-angle triangle pattern where each row displays numbers incrementally from 1 to the row number. It utilizes nested “for” loops to control the number of rows and the numbers displayed in each row, creating the desired pattern.
Visual Presentation:
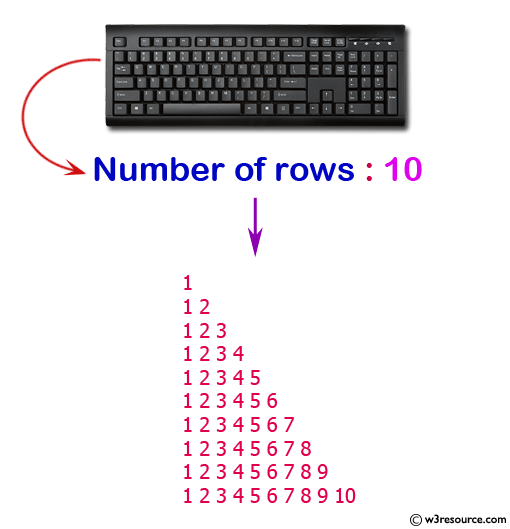
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main() {
int i, j, rows; // Declare variables 'i' and 'j' for loop counters, 'rows' for user input.
printf("Input number of rows : "); // Print a message to prompt user input.
scanf("%d", &rows); // Read the value of 'rows' from the user.
for (i = 1; i <= rows; i++) { // Start a loop to generate rows.
for (j = 1; j <= i; j++) // Nested loop to print numbers based on the current row.
printf("%d", j); // Print the value of 'j'.
printf("\n"); // Move to the next line for the next row.
}
}
Output:
Input number of rows : 10 1 12 123 1234 12345 123456 1234567 12345678 123456789 12345678910
Explanation:
for (i = 1; i <= rows; i++) { for (j = 1; j <= i; j++) printf("%d", j); printf("\n"); }
In the above loop, the variable i is initialized to 1, and the loop will continue as long as i is less than or equal to the value of variable 'rows'. In each iteration of the outer loop, another loop is started with variable j, initialized to 1, and it will continue as long as j is less than or equal to the value of i.
In each iteration of the inner loop, the printf() function will print the value of j to the console. This value will be printed j times, as the value of j is increasing by 1 in each iteration of the inner loop.
After the inner loop completes, the outer loop will print a newline character (\n) to create a new line.
Finally, the outer loop will increment the value of i by 1, and the process will repeat until the condition i<=rows is no longer true.
Flowchart:
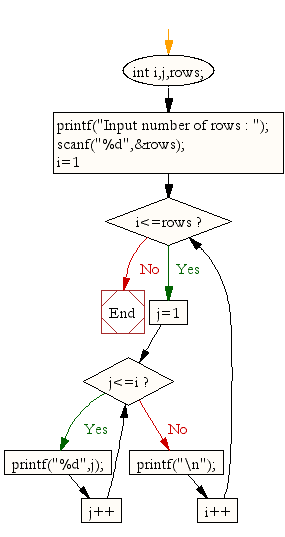
C Programming Code Editor:
Previous: Write a program in C to display specified Pattern.
Next: Write a program in C to make such a pattern like right angle triangle with a number which will repeat a number in a row.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics