C Exercises: Compute the area of a circle given its radius using inline function
C inline function: Exercise-1 with Solution
Write a C program to compute the area of a circle given its radius using inline functions.
Sample Solution:
C Code:
#include <stdio.h>
// Inline function to compute area of a circle
inline double circle_area(double radius) {
return 3.14159265358979323846 * radius * radius;
}
int main() {
double radius = 5.0;
printf("The area of the circle (radius = %f) is %f", radius, circle_area(radius));
radius = 12.0;
printf("\nThe area of the circle (radius = %f) is %f", radius, circle_area(radius));
return 0;
}
Sample Output:
The area of the circle (radius = 5.000000) is 78.539816 The area of the circle (radius = 12.000000) is 452.389342
Explanation:
The said program is an inline function that computes the area of a circle given its radius. The formula for circle area is πr², where r is the radius. In the inline function, pi is taken as a constant, and the radius is passed as an argument to the function. The function then computes the area using the formula and returns the result. Since it is an inline function, it is inserted directly into the code where it is called. This can improve performance compared to regular function calls.
Flowchart:
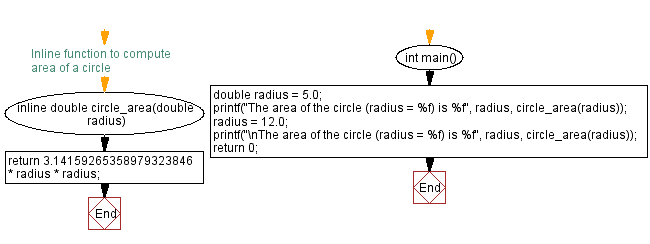
C Programming Code Editor:
Previous: C Inline function Exercises Home.
Next: Compute absolute difference between two integers using inline function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/inline_function/c-inline-function-exercise-1.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics