C Exercises: Compute absolute difference between two integers using inline function
2. Absolute Difference Inline Variants
Write a C program to compute absolute difference between two integers using inline functions.
Sample Solution:
C Code:
#include <stdio.h>
inline int absolute_difference(int a, int b) {
return (a > b) ? (a - b) : (b - a);
}
int main() {
int x = 100, y = 200;
int diff = absolute_difference(x, y);
printf("Absolute difference between %d and %d is %d\n", x, y, diff);
printf("\nAbsolute difference between %d and %d is %d\n", y, x, diff);
return 0;
}
Sample Output:
Absolute difference between 100 and 200 is 100 Absolute difference between 200 and 100 is 100
Explanation:
The above program is an inline C function that computes the absolute difference between two integers. The absolute difference is the positive value of the difference between the two integers, regardless of which one is the higher. The function takes two integer parameters and returns their absolute difference as an integer value. By using an inline function, the compiler can replace the function call with the actual function code during compilation. This results in faster program execution.
Flowchart:
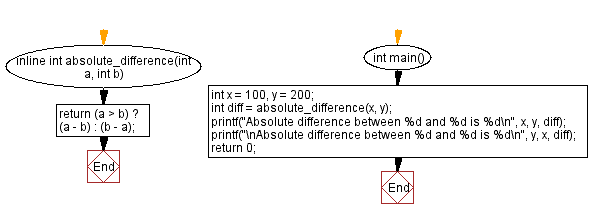
For more Practice: Solve these Related Problems:
- Write a C program to compute the absolute difference between two floating-point numbers using an inline function.
- Write a C program to compute the absolute difference of two numbers and then add a constant offset using an inline function.
- Write a C program to calculate the absolute difference between two large integers using an inline function with error checking.
- Write a C program to determine the absolute difference between two numbers passed by reference using an inline function.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Compute the area of a circle given its radius using inline function.
Next: Compute the minimum of two integers using inline function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.