C Exercises: Compute the minimum of two integers using inline function
C inline function: Exercise-3 with Solution
Write a C program to compute the minimum of two integers using inline function.
Sample Solution:
C Code:
#include <stdio.h>
inline int min(int x, int y) {
return (x < y) ? x : y;
}
int main() {
int a = 110, b = 120;
printf("Minimum of %d and %d is %d\n", a, b, min(a, b));
a = -110, b = -120;
printf("Minimum of %d and %d is %d\n", a, b, min(a, b));
return 0;
}
Sample Output:
Minimum of 110 and 120 is 110 Minimum of -110 and -120 is -120
Explanation:
In the above program, we define an inline function min() that takes two integers x and y as parameters and returns the minimum value between them. We use the ternary operator ? : to check which value is smaller and return it.
In the main function, we declare two integers a and b and assign them 110 and 120 and -110 and -120 respectively. We then call the min() function with a and b as arguments and print the result using the printf() function.
Flowchart:
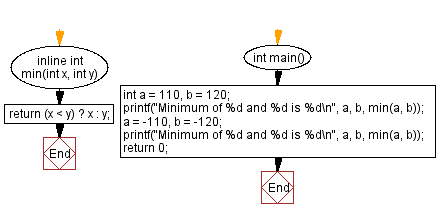
C Programming Code Editor:
Previous: Compute absolute difference between two integers using inline function.
Next: Compute the maximum of two integers using inline function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics