C Exercises: Compute the maximum of two integers using inline function
4. Maximum of Two Integers Inline Variants
Write a C program to compute the maximum of two integers using inline function.
Sample Solution:
C Code:
#include <stdio.h>
inline int max(int x, int y) {
return x > y ? x : y;
}
int main() {
int a = 110, b = 120;
printf("Maximum of %d and %d is %d\n", a, b, max(a, b));
a = -110, b = -120;
printf("Maximum of %d and %d is %d\n", a, b, max(a, b));
return 0;
}
Sample Output:
Maximum of 110 and 120 is 120 Maximum of -110 and -120 is -110
Explanation:
In the above program, we define an inline function max() that takes two integers x and y as parameters and returns the maximum value between them. We use the ternary operator ? : to check which value is maximum and return it.
In the main function, we declare two integers a and b and assign them 110 and 120 and -110 and -120 respectively. We then call the max() function with a and b as arguments and print the result using the printf() function.
Flowchart:
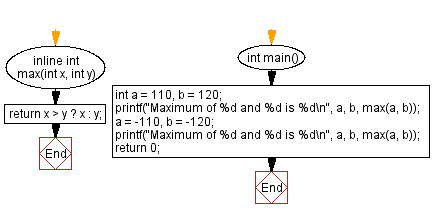
For more Practice: Solve these Related Problems:
- Write a C program to compute the maximum of three integers using an inline function.
- Write a C program to find the maximum element in an array of integers using an inline function.
- Write a C program to determine the maximum of two floating-point numbers using an inline function.
- Write a C program to compare two strings and return the lexicographically larger string using an inline function.
C Programming Code Editor:
Previous: Compute the minimum of two integers using inline function.
Next: Compute the length of a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.