C Exercises: Compute the length of a given string
5. String Length Inline Variants
Write a C program to compute the length of a given string using inline function.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
inline int string_length(char * str) {
int len = 0;
while ( * str++) {
len++;
}
return len;
}
int main() {
char str[] = "w3resource.com";
int len = string_length(str);
printf("The length of the string '%s' is %d\n", str, len);
char str1[] = "Learn C Programming";
len = string_length(str1);
printf("\nThe length of the string '%s' is %d\n", str1, len);
return 0;
}
Sample Output:
The length of the string 'w3resource.com' is 14 The length of the string 'Learn C Programming' is 19
Explanation:
In this exercise, the string_length function is defined as an inline function using the inline keyword before the function signature. The function takes a pointer to a character array (string) as its argument, and returns the string length as an integer.
The main function in this example initializes a character array with a string. It calls the string_length function to compute the string length, and prints the result to the console using the printf() function.
Flowchart:
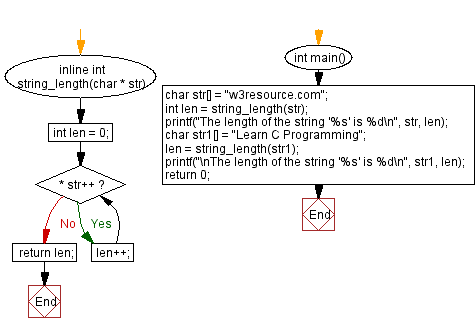
For more Practice: Solve these Related Problems:
- Write a C program to compute the length of a string ignoring whitespace using an inline function.
- Write a C program to calculate the length of a string using pointer arithmetic in an inline function.
- Write a C program to count only alphabetic characters in a string using an inline function.
- Write a C program to determine the length of a string and verify it against a user-specified value using an inline function.
C Programming Code Editor:
Previous: Compute the maximum of two integers using inline function.
Next: Convert a temperature from Celsius to Fahrenheit and vice versa.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.