C Exercises: Convert a temperature from Celsius to Fahrenheit and vice versa
6. Temperature Conversion Inline Variants
Write a C program to convert a temperature from Celsius to Fahrenheit and vice versa using an inline function.
Sample Solution:
C Code:
#include <stdio.h>
inline float celsius_To_Fahrenheit(float celsius) {
return (celsius * 9 / 5) + 32;
}
inline float fahrenheit_To_Celsius(float fahrenheit) {
return (fahrenheit - 32) * 5 / 9;
}
int main() {
float celsius = 32;
float fahrenheit = 89.60;
printf("%.2f Celsius is equal to %.2f Fahrenheit\n", celsius, celsius_To_Fahrenheit(celsius));
printf("\n%.2f Fahrenheit is equal to %.2f Celsius\n", fahrenheit,
fahrenheit_To_Celsius(fahrenheit));
return 0;
}
Sample Output:
32.00 Celsius is equal to 89.60 Fahrenheit 89.60 Fahrenheit is equal to 32.00 Celsius
Explanation:
The above program defines two inline functions "Celsius_To_Fahrenheit()" and "Fahrenheit_To_Celsius()" to convert temperature values between Celsius and Fahrenheit. The functions use the formulas (Celsius * 9/5) + 32 and (Fahrenheit - 32) * 5/9, respectively. The main() function calls these functions and prints the results to the console.
Flowchart:
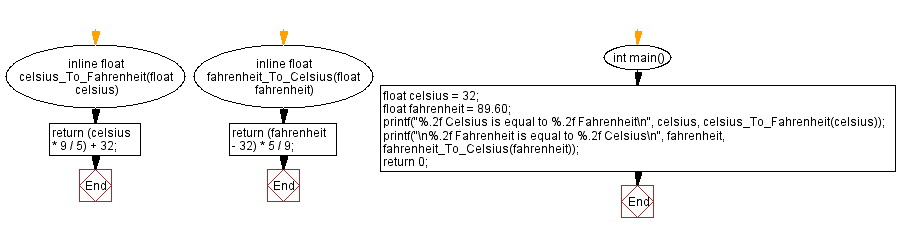
For more Practice: Solve these Related Problems:
- Write a C program to convert Fahrenheit to Celsius using an inline function.
- Write a C program to convert Celsius to Kelvin using an inline function.
- Write a C program to perform dual temperature conversions (Celsius to Fahrenheit and vice versa) in a single inline function.
- Write a C program to convert a temperature value and round the result to the nearest integer using an inline function.
C Programming Code Editor:
Previous: Compute the length of a given string.
Next: Count the number of vowels in a given string using an inline function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.