C Programming: Count the digits in a number that divide it
C Programming Mathematics: Exercise-33 with Solution
Write a C program to count the digits in a given number that divide it.
Example:
Input (integer value) = 9
Digit of the said number is 9
9 divides 9
So, Output = 1
Input (integer value) = 27
Digits of the said number are 2, 7
2 cannot divides 27
7 cannot divides 27
So, Output = 0
Input (integer value) = 2245
Digits of the said number are 2, 2, 4, 5
2 cannot divides 2245
2 cannot divides 2245
4 cannot divides 2245
5 divides 2245
So, Output = 0
Test Data:
(9) -> 1
(27) -> 0
(145) -> 2
(2245) -> 1
(2222) -> 4
Sample Solution:
C Code:
#include <stdio.h>
// Function to count the number of digits in 'n' that evenly divide 'n'
int test(int n){
int temp = n; // Temporary variable to store 'n'
int result = 0; // Variable to store the count of digits that divide 'n'
// Loop to iterate through each digit of 'n'
while(temp != 0){
int d = temp % 10; // Extract the rightmost digit of 'temp'
temp /= 10; // Move to the next digit by dividing 'temp' by 10
// Check if 'n' is divisible by the extracted digit 'd'
if(n % d == 0)
result++; // Increment the count if 'n' is divisible by 'd'
}
return result; // Return the total count of digits that divide 'n'
}
// Main function
int main(void) {
int n = 9;
printf("n = %d", n);
printf("\nNumber of digits in the said number that divide it = %d", test(n));
// Displaying results for different numbers
n = 27;
printf("\nn = %d", n);
printf("\nNumber of digits in the said number that divide it = %d", test(n));
n = 145;
printf("\nn = %d", n);
printf("\nNumber of digits in the said number that divide it = %d", test(n));
n = 2245;
printf("\nn = %d", n);
printf("\nNumber of digits in the said number that divide it = %d", test(n));
n = 2222;
printf("\nn = %d", n);
printf("\nNumber of digits in the said number that divide it = %d", test(n));
}
Sample Output:
n = 9 Number of digits in the said number that divide it = 1 n = 27 Number of digits in the said number that divide it = 0 n = 145 Number of digits in the said number that divide it = 2 n = 2245 Number of digits in the said number that divide it = 1 n = 2222 Number of digits in the said number that divide it = 4
Flowchart:
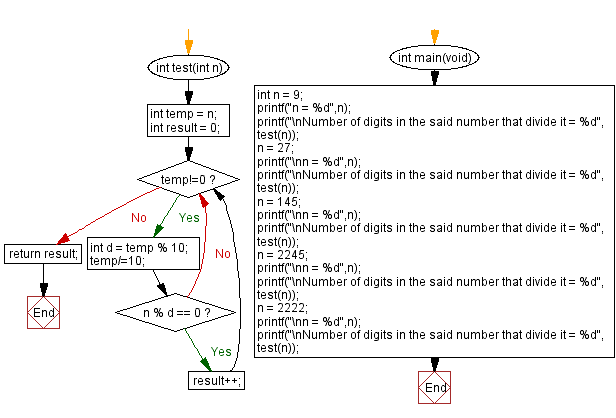
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Number sums and their reverses.
Next: Kth smallest number in a multiplication table.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/math/c-math-exercise-33.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics