C Exercises: Demonstrate the use of & and * operator
3. Use of & and * Operators
Write a program in C to demonstrate the use of the &(address of) and *(value at address) operators.
Visual Presentation:
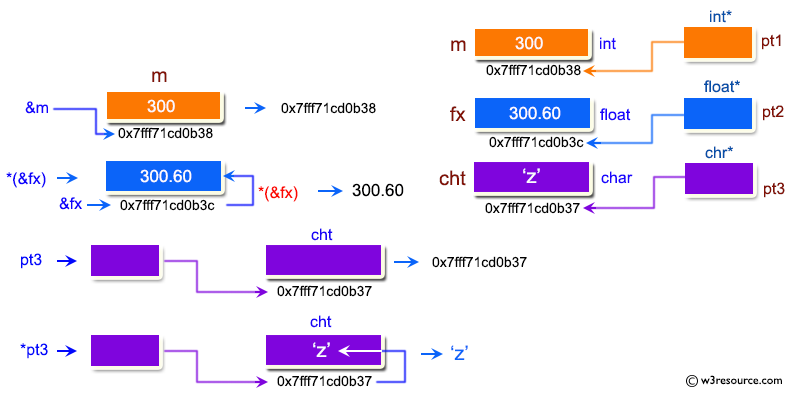
Sample Solution:
C Code:
#include <stdio.h>
int main()
{
int m = 300; // Declare and initialize an integer variable m
float fx = 300.60; // Declare and initialize a float variable fx
char cht = 'z'; // Declare and initialize a char variable cht
printf("\n\n Pointer : Demonstrate the use of & and * operator :\n");
printf("--------------------------------------------------------\n");
int *pt1; // Declare an integer pointer pt1
float *pt2; // Declare a float pointer pt2
char *pt3; // Declare a char pointer pt3
pt1 = &m; // Assign the address of m to pointer pt1
pt2 = &fx; // Assign the address of fx to pointer pt2
pt3 = &cht; // Assign the address of cht to pointer pt3
printf(" m = %d\n", m); // Print the value of m
printf(" fx = %f\n", fx); // Print the value of fx
printf(" cht = %c\n", cht); // Print the value of cht
printf("\n Using & operator :\n");
printf("-----------------------\n");
printf(" address of m = %p\n", &m); // Print the address of m
printf(" address of fx = %p\n", &fx); // Print the address of fx
printf(" address of cht = %p\n", &cht); // Print the address of cht
printf("\n Using & and * operator :\n");
printf("-----------------------------\n");
printf(" value at address of m = %d\n", *(&m)); // Print the value at the address of m
printf(" value at address of fx = %f\n", *(&fx)); // Print the value at the address of fx
printf(" value at address of cht = %c\n", *(&cht)); // Print the value at the address of cht
printf("\n Using only pointer variable :\n");
printf("----------------------------------\n");
printf(" address of m = %p\n", pt1); // Print the address stored in pt1
printf(" address of fx = %p\n", pt2); // Print the address stored in pt2
printf(" address of cht = %p\n", pt3); // Print the address stored in pt3
printf("\n Using only pointer operator :\n");
printf("----------------------------------\n");
printf(" value at address of m = %d\n", *pt1); // Print the value pointed by pt1
printf(" value at address of fx= %f\n", *pt2); // Print the value pointed by pt2
printf(" value at address of cht= %c\n\n", *pt3); // Print the value pointed by pt3
}
Sample Output:
Pointer : Demonstrate the use of & and * operator : -------------------------------------------------------- m = 300 fx = 300.600006 cht = z Using & operator : ----------------------- address of m = 0x7fff71cd0b38 address of fx = 0x7fff71cd0b3c address of cht = 0x7fff71cd0b37 Using & and * operator : ----------------------------- value at address of m = 300 value at address of fx = 300.600006 value at address of cht = z Using only pointer variable : ---------------------------------- address of m = 0x7fff71cd0b38 address of fx = 0x7fff71cd0b3c address of cht = 0x7fff71cd0b37 Using only pointer operator : ---------------------------------- value at address of m = 300 value at address of fx= 300.600006 value at address of cht= z
Flowchart:
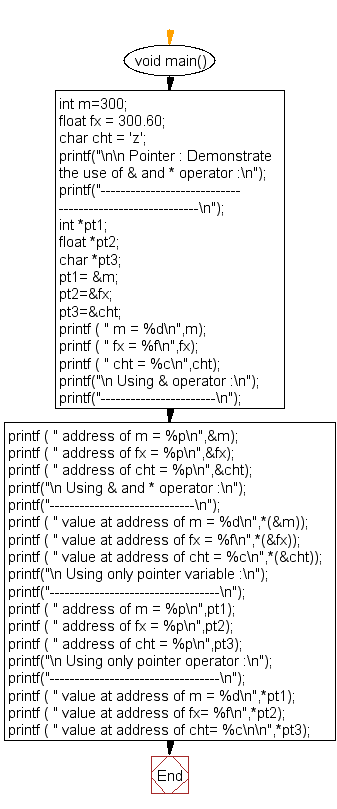
For more Practice: Solve these Related Problems:
- Write a C program to input an integer, then display its address, value using & and *, and demonstrate modifying the value via pointer.
- Write a C program to declare several variables, print their addresses using &, and then use * to print the values stored at these addresses.
- Write a C program to combine the use of & and * operators to swap the values of two variables without using a temporary variable.
- Write a C program to create a pointer, assign it the address of a variable, and then use both operators to increment the variable’s value and display the results.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a program in C to demonstrate how to handle the pointers in the program.
Next: Write a program in C to add two numbers using pointers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.