Book details in C: Finding most expensive and lowest priced books
C programming related to structures: Exercise-3 with Solution
Create a structure named Book to store book details like title, author, and price. Write a C program to input details for three books, find the most expensive and the lowest priced books, and display their information.
Sample Solution:
C Code:
#include <stdio.h>
#include <float.h> // Include for FLT_MAX and FLT_MIN constants
// Define the structure "Book"
struct Book {
char title[100];
char author[100];
float price;
};
int main() {
// Declare variables to store details for three books
struct Book book1, book2, book3;
// Input details for the first book
printf("Input details for Book 1:\n");
printf("Title: ");
scanf("%s", book1.title); // Assuming titles do not contain spaces
printf("Author: ");
scanf("%s", book1.author); // Assuming authors do not contain spaces
printf("Price: ");
scanf("%f", &book1.price);
// Input details for the second book
printf("\nInput details for Book 2:\n");
printf("Title: ");
scanf("%s", book2.title);
printf("Author: ");
scanf("%s", book2.author);
printf("Price: ");
scanf("%f", &book2.price);
// Input details for the third book
printf("\nInput details for Book 3:\n");
printf("Title: ");
scanf("%s", book3.title);
printf("Author: ");
scanf("%s", book3.author);
printf("Price: ");
scanf("%f", &book3.price);
// Find the most expensive book
struct Book mostExpensive;
if (book1.price >= book2.price && book1.price >= book3.price) {
mostExpensive = book1;
} else if (book2.price >= book1.price && book2.price >= book3.price) {
mostExpensive = book2;
} else {
mostExpensive = book3;
}
// Find the lowest priced book
struct Book lowestPriced;
if (book1.price <= book2.price && book1.price <= book3.price) {
lowestPriced = book1;
} else if (book2.price <= book1.price && book2.price <= book3.price) {
lowestPriced = book2;
} else {
lowestPriced = book3;
}
// Display information for the most expensive book
printf("\nMost Expensive Book:\n");
printf("Title: %s\n", mostExpensive.title);
printf("Author: %s\n", mostExpensive.author);
printf("Price: %.2f\n", mostExpensive.price);
// Display information for the lowest priced book
printf("\nLowest Priced Book:\n");
printf("Title: %s\n", lowestPriced.title);
printf("Author: %s\n", lowestPriced.author);
printf("Price: %.2f\n", lowestPriced.price);
return 0;
}
Output:
Input details for Book 1: Title: Book-1 Author: Author-1 Price: 100 Input details for Book 2: Title: Book-2 Author: Author-2 Price: 120 Input details for Book 3: Title: Book-3 Author: Author-3 Price: 111 Most Expensive Book: Title: Book-2 Author: Author-2 Price: 120.00 Lowest Priced Book: Title: Book-1 Author: Author-1 Price: 100.00
Explanation:
In the exercise above,
- The Book structure is defined with members title, author, and price.
- Three variables (book1, book2, and book3) are declared to store details for three books.
- The program prompts the user to input details for each book.
- It then finds the most expensive and lowest priced books.
- Finally, it displays information for both the most expensive and lowest priced books.
Flowchart:
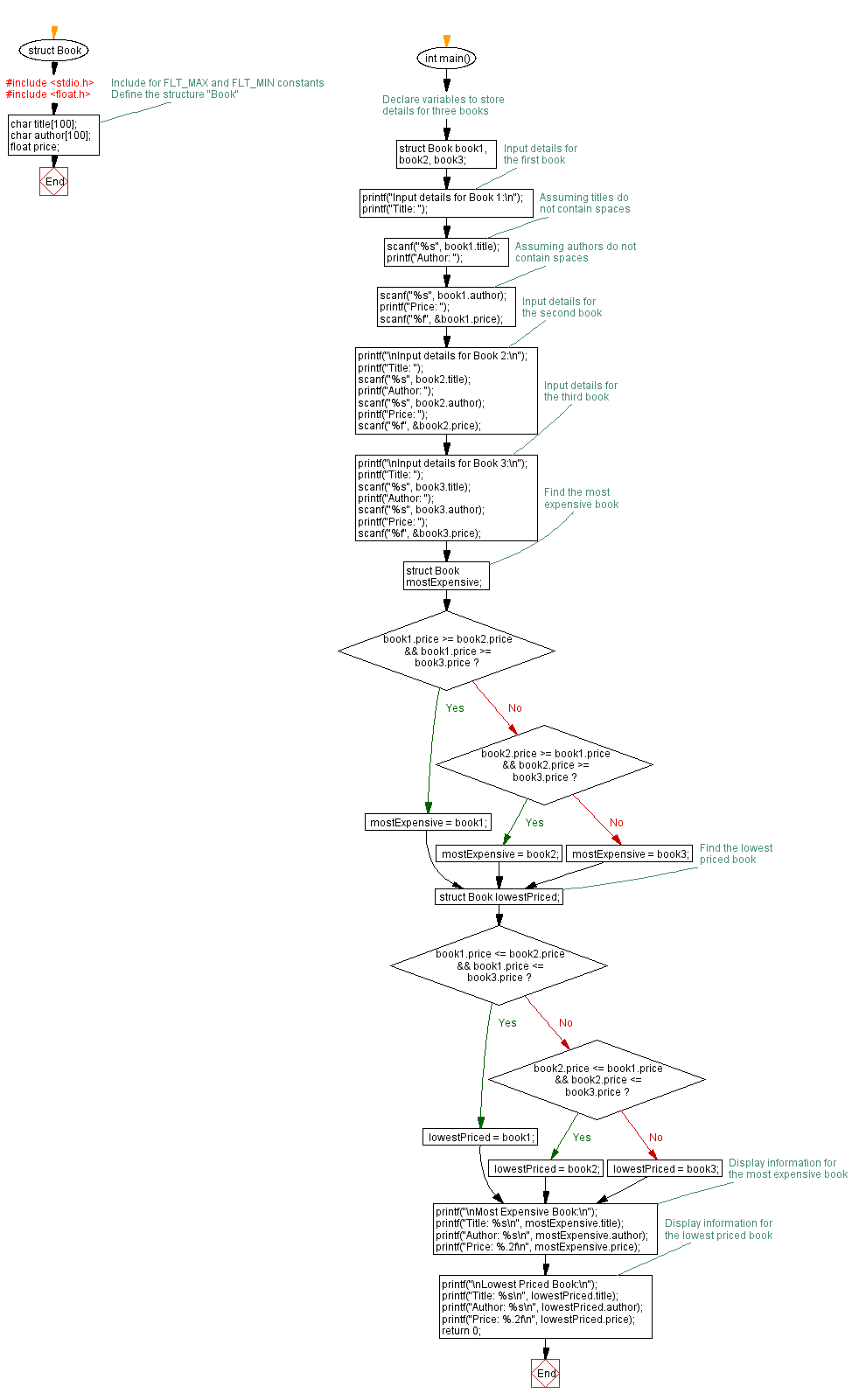
C Programming Code Editor:
Previous: Adding two times using time Structure in C: Example and explanation.
Next: C Program Structure: Circle area and perimeter calculation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/structure/c-structure-exercises-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics