Adding two times using time Structure in C: Example and explanation
2. Time Structure Calculations
Define a structure named Time with members hours, minutes, and seconds. Write a C program to input two times, add them, and display the result in proper time format.
Sample Solution:
C Code:
#include <stdio.h>
// Define the structure "Time"
struct Time {
int hours;
int minutes;
int seconds;
};
int main() {
// Declare variables to store two times and the result
struct Time time1, time2, result;
// Input time1
printf("Input the first time (hours minutes seconds): ");
scanf("%d %d %d", &time1.hours, &time1.minutes, &time1.seconds);
// Input time2
printf("Input the second time (hours minutes seconds): ");
scanf("%d %d %d", &time2.hours, &time2.minutes, &time2.seconds);
// Add the two times
result.seconds = time1.seconds + time2.seconds;
result.minutes = time1.minutes + time2.minutes + result.seconds / 60;
result.hours = time1.hours + time2.hours + result.minutes / 60;
// Adjust minutes and seconds
result.minutes %= 60;
result.seconds %= 60;
// Display the result
printf("\nResultant Time: %02d:%02d:%02d\n", result.hours, result.minutes, result.seconds);
return 0;
}
Output:
Input the first time (hours minutes seconds): 7 12 20 Input the second time (hours minutes seconds): 8 10 55 Resultant Time: 15:23:15
Explanation:
In the exercise above,
- The Time structure is defined with members hours, minutes, and seconds.
- Three variables (time1, time2, and result) are declared to store the input times and the result of the addition.
- The user is prompted to input two times using the format hours minutes seconds.
- The program adds the two times, adjusting for overflow in minutes and seconds.
- The result is displayed in proper time format using %02d to ensure two-digit representation for hours, minutes, and seconds.
Flowchart:
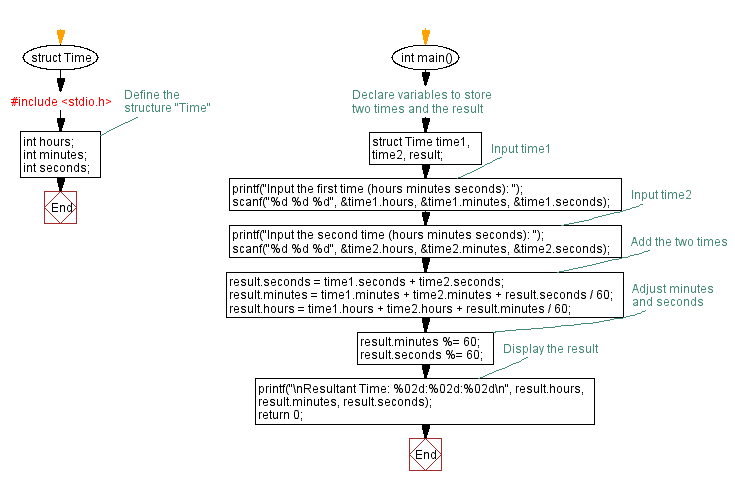
For more Practice: Solve these Related Problems:
- Write a C program to input two times, subtract the earlier from the later, and display the time difference in proper format.
- Write a C program to convert two input times into seconds, then output both the sum and difference in hours, minutes, and seconds.
- Write a C program to input an array of Time structures, sort them chronologically, and display the sorted times.
- Write a C program to add multiple time values (from an array of Time structures) and display the cumulative time in proper time format.
C Programming Code Editor:
Previous: Student Structure in C: Input, display, and average marks calculation.
Next: Book details in C: Finding most expensive and lowest priced books.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.