Student Structure in C: Input, display, and average marks calculation
1. Student Structure Operations
Create a structure called "Student" with members name, age, and total marks. Write a C program to input data for two students, display their information, and find the average of total marks.
Sample Solution:
C Code:
#include <stdio.h>
// Define the structure "Student"
struct Student {
char name[50];
int age;
float totalMarks;
};
int main() {
// Declare variables to store information for two students
struct Student student1, student2;
// Input data for the first student
printf("Input details for Student 1:\n");
printf("Name: ");
scanf("%s", student1.name); // Assuming names do not contain spaces
printf("Age: ");
scanf("%d", &student1.age);
printf("Total Marks: ");
scanf("%f", &student1.totalMarks);
// Input data for the second student
printf("\nInput details for Student 2:\n");
printf("Name: ");
scanf("%s", student2.name); // Assuming names do not contain spaces
printf("Age: ");
scanf("%d", &student2.age);
printf("Total Marks: ");
scanf("%f", &student2.totalMarks);
// Display information for both students
printf("\nStudent 1 Information:\n");
printf("Name: %s\n", student1.name);
printf("Age: %d\n", student1.age);
printf("Total Marks: %.2f\n", student1.totalMarks);
printf("\nStudent 2 Information:\n");
printf("Name: %s\n", student2.name);
printf("Age: %d\n", student2.age);
printf("Total Marks: %.2f\n", student2.totalMarks);
// Calculate and display the average total marks
float averageMarks = (student1.totalMarks + student2.totalMarks) / 2;
printf("\nAverage Total Marks: %.2f\n", averageMarks);
return 0;
}
Output:
Input details for Student 1: Name: Climacus Age: 14 Total Marks: 189 Input details for Student 2: Name: Meredith Age: 14 Total Marks: 192 Student 1 Information: Name: Climacus Age: 14 Total Marks: 189.00 Student 2 Information: Name: Meredith Age: 14 Total Marks: 192.00 Average Total Marks: 190.50
Flowchart:
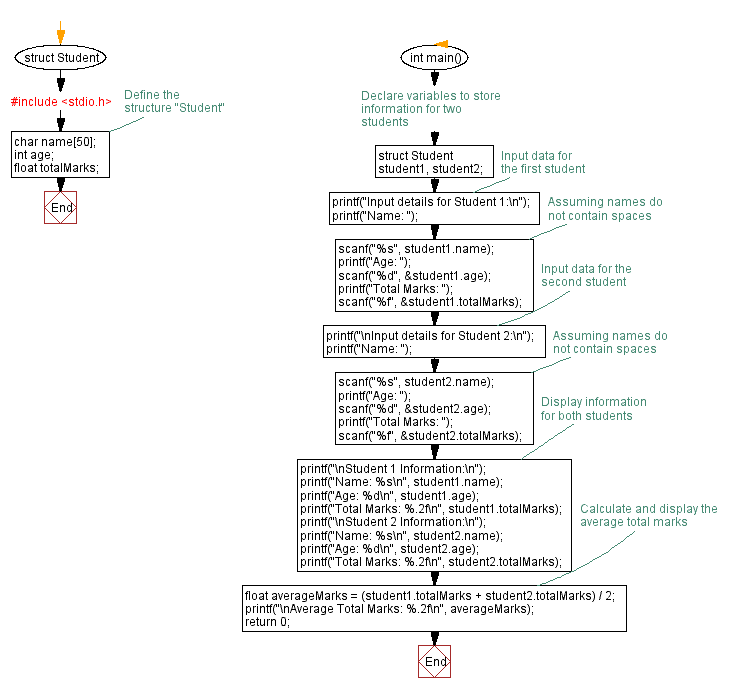
For more Practice: Solve these Related Problems:
- Write a C program to input details for three students, sort them by total marks, and display the sorted list along with the average marks.
- Write a C program to input data for two students and then update the student with lower marks by adding bonus points before displaying their information.
- Write a C program to store student details in an array of structures, then compute and display the highest, lowest, and average total marks.
- Write a C program to input a student’s data and display the information with the student’s name reversed (i.e. reverse the characters in the name).
C Programming Code Editor:
Previous: C Programming Structure Exercises and Solutions Home.
Next: Adding two times using time Structure in C: Example and explanation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.