C Exercises: Implement the printf() function
C Variadic function: Exercise-7 with Solution
Write a C program to implement the printf() function using variadic functions.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdarg.h>
void printValues(char * format, ...) {
va_list argList;
va_start(argList, format);
printf("The values are: ");
while ( * format != '\0') {
if ( * format == 'd') {
int val = va_arg(argList, int);
printf("%d ", val);
}
format++;
}
printf("\n");
va_end(argList);
}
int main() {
int x = 10;
int y = 20;
int z = 30;
printValues("ddd", x, y, z);
return 0;
}
Sample Output:
The values are: 10 20 30
Explanation:
This above program defines the function printValues () that takes a string format and a variable number of arguments. Inside the function, we use the va_list, va_start(), va_arg(), and va_end() macros from the stdarg.h library to iterate over the variable arguments.
We then loop through each character in the format string and check if it is a % character. If it is, we use va_arg() to get the next argument as an integer and print it with printf(). If it is not a % character, we print it using printf().
Flowchart:
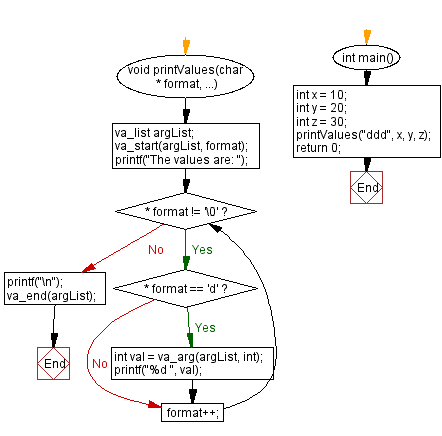
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Average of a variable number.
Next: Sort a variable number of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics