C Exercises: Average of a variable number
6. Variadic Average Function Challenges
Write a C program to find the average of a variable number of doubles passed as arguments to a function using variadic functions.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdarg.h>
double calculate_average(int num, ...) {
double sum = 0;
int i;
va_list arguments;
va_start(arguments, num);
for (i = 0; i < num; i++) {
sum += va_arg(arguments, double);
}
va_end(arguments);
return sum / num;
}
int main() {
double avg = calculate_average(4, 10.2, 20.3, 30.4, 40.5);
printf("The average is: %f\n", avg);
return 0;
}
Sample Output:
The average is: 25.350000
Explanation:
In the above program, we define a function called "calculate_average()" that accepts a variable number of arguments. It starts by initializing a va_list object called arguments with va_start. This takes the va_list object and the number of arguments passed. We then loop through the arguments, adding them to a running total in the sum variable. This is done using the va_arg function, which takes the va_list object and the type of argument we want to retrieve. Finally, we use va_end to free the va_list object and return the average of the numbers.
In the main function, we call the “calculate_average()” function with four arguments, and print out the result. This program can be used to find the average of any number of doubles passed as arguments.
Flowchart:
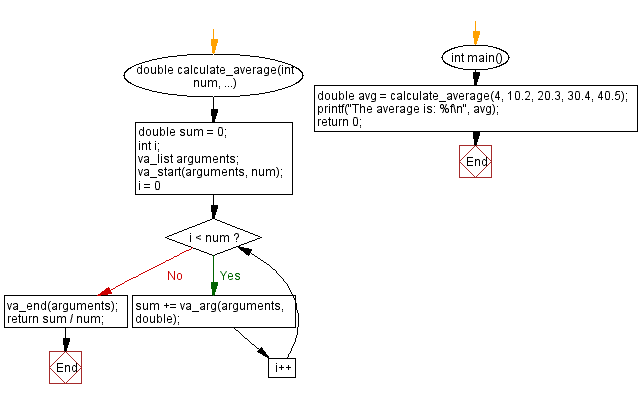
For more Practice: Solve these Related Problems:
- Write a C program to compute the average of a variable number of doubles using a variadic function, ensuring proper type handling.
- Write a C program to implement a variadic function that calculates both the average and the standard deviation of doubles.
- Write a C program to compute the average from variadic double arguments and gracefully handle the case when no values are provided.
- Write a C program to calculate the average of a series of double values using a variadic function and output the result with high precision.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Characters in a variable number of strings.
Next: Implement the printf() function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.