C Exercises: Characters in a variable number of strings
C Variadic function: Exercise-5 with Solution
Write a C program to count the number of characters in a variable number of strings passed as arguments to a function using variadic functions.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdarg.h>
int count_chars(int ctr, ...) {
va_list args;
int i, total = 0;
char * str;
va_start(args, ctr);
for (i = 0; i < ctr; i++) {
str = va_arg(args, char * );
while ( * str != '\0') {
total++;
str++;
}
}
va_end(args);
return total;
}
int main() {
int ctr = 3;
char * str1 = "w3resource";
char * str2 = ".";
char * str3 = "com";
printf("Original strings:");
printf("\nString-1: %s", str1);
printf("\nString-2: %s", str2);
printf("\nString-3: %s", str3);
int num_chars = count_chars(ctr, str1, str2, str3);
printf("\nThe total number of characters is %d\n", num_chars);
return 0;
}
Sample Output:
Original strings: String-1: w3resource String-2: . String-3: com The total number of characters is 14
Explanation:
In the above exercise, the count_chars() function takes two arguments number of strings and a variable number of arguments using the ... syntax. The va_list type and va_start(), va_arg(), and va_end() macros are used to access the arguments passed to the function. The function loops through each string, counting the number of characters in each one, and returns the total count.
In the main() function, the count_chars() function is called with three strings as arguments, and the result is printed to the console.
Flowchart:
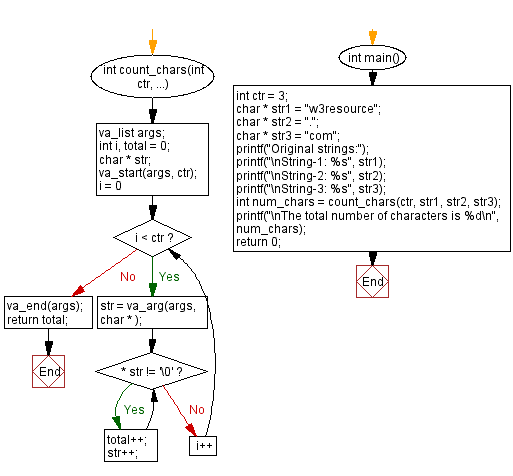
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Concatenate a variable number of strings.
Next: Average of a variable number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics