C Exercises: Concatenate a variable number of strings
4. Variadic String Concatenation Challenges
Write a C program to concatenate a variable number of strings passed as arguments to a function using variadic functions.
Sample Solution:
C Code:
#include <stdarg.h>
#include <string.h>
void concat(char * result, int num, ...) {
va_list args;
va_start(args, num);
for (int i = 0; i < num; i++) {
strcat(result, va_arg(args, char * ));
}
va_end(args);
}
int main() {
char str1[100] = "w3resource";
char str2[100] = ".";
char str3[100] = "com";
printf("Original strings:");
printf("\nString-1: %s", str1);
printf("\nString-2: %s", str2);
printf("\nString-3: %s", str3);
concat(str1, 2, str2, str3);
printf("\nConcatenate said strings: %s", str1);
return 0;
}
Sample Output:
Original strings: String-1: w3resource String-2: . String-3: com Concatenate said strings: w3resource.com
Explanation:
In the above program, the concat() function takes a variable number of arguments using the ... syntax. The ‘num’ parameter indicates the number of arguments that will follow. Inside the function, the va_list type is used to declare a list of arguments, and va_start() is called to initialize the list with the first argument. The va_arg() function is then used to access each of the subsequent arguments in turn.
The strcat() function is used to concatenate the strings together, and the resulting string is stored in the result parameter. Finally, va_end() is called to clean up the argument list.
In the main() function, three strings are declared and initialized. The concat() function is then called with the three strings as arguments, as well as a fourth string literal. The resulting string is then printed to the console.
Flowchart:
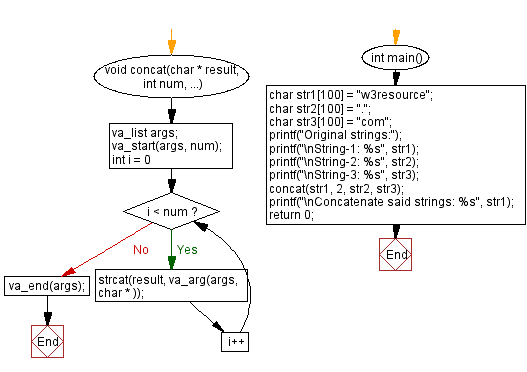
For more Practice: Solve these Related Problems:
- Write a C program to concatenate a variable number of strings using a variadic function and dynamic memory allocation.
- Write a C program to join multiple strings with a specified delimiter using a variadic function.
- Write a C program to concatenate variable strings and then convert the result to uppercase within the same variadic function.
- Write a C program to merge a variable number of strings using a variadic function and subsequently reverse the concatenated string.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Maximum and minimum of a variable number of integers.
Next: Characters in a variable number of strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.