C Exercises: Maximum and minimum of a variable number of integers
C Variadic function: Exercise-3 with Solution
Write a C program to find the maximum and minimum values of a variable number of integers passed as arguments to a function using variadic functions.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdarg.h>
int max(int count, ...) {
va_list args;
int i, max, num;
/* Initialize the argument list */
va_start(args, count);
/* Get the first argument and set it as the maximum value */
max = va_arg(args, int);
/* Compare the remaining arguments to the current maximum value */
for (i = 1; i < count; i++) {
num = va_arg(args, int);
if (num > max) {
max = num;
}
}
/* Clean up the argument list */
va_end(args);
return max;
}
int min(int count, ...) {
va_list args;
int i, min, num;
/* Initialize the argument list */
va_start(args, count);
/* Get the first argument and set it as the minimum value */
min = va_arg(args, int);
/* Compare the remaining arguments to the current minimum value */
for (i = 1; i < count; i++) {
num = va_arg(args, int);
if (num < min) {
min = num;
}
}
/* Clean up the argument list */
va_end(args);
return min;
}
int main() {
int max_val = max(6, 1, 5, 7, 2, 9, 0);
int min_val = min(6, 1, 5, 7, 2, 9, 0);
printf("Maximum value: %d\n", max_val);
printf("Minimum value: %d\n", min_val);
return 0;
}
Sample Output:
Maximum value: 9 Minimum value: 0
Explanation:
In the above code, the max() and min() functions take a variable number of arguments using the ... syntax. The va_list data type is used to initialize and access the argument list, and the va_start(), va_arg(), and va_end() macros are used to work with the list.
The max() function finds the maximum value by comparing each argument to the current maximum value, and the min() function finds the minimum value in a similar way. The main() function calls these functions with a list of integers and prints out the results.
Flowchart:
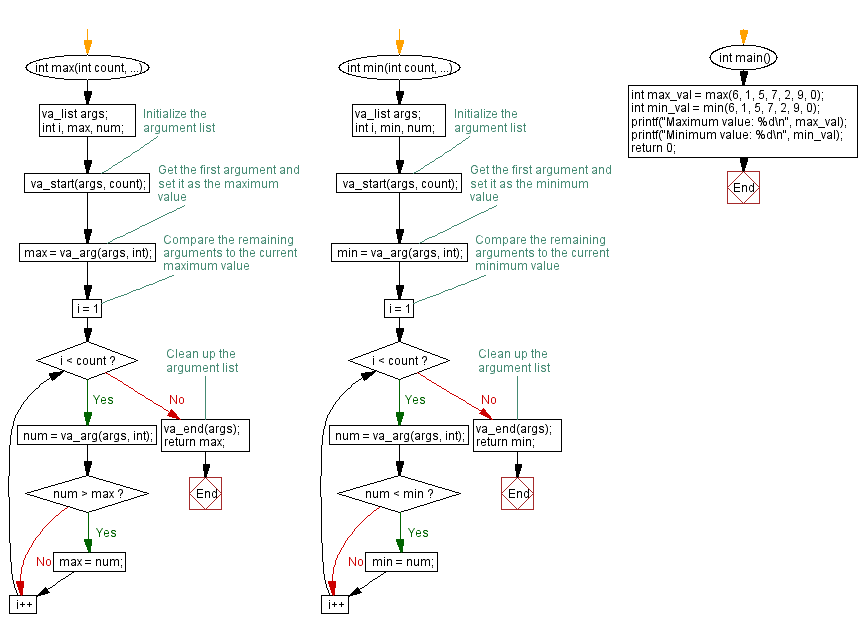
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Product of a variable number of integers.
Next: Concatenate a variable number of strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/variadic/c-variadic-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics