C Exercises: Product of a variable number of integers
2. Variadic Product Function Challenges
Write a C program to find the product of a variable number of integers passed as arguments to a function using variadic functions.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdarg.h>
int multiply(int count, ...) {
int result = 1;
va_list args;
va_start(args, count);
for (int i = 0; i < count; i++) {
int num = va_arg(args, int);
result *= num;
}
va_end(args);
return result;
}
int main() {
int p1 = multiply(4, 2, 3, 4, 5);
int p2 = multiply(3, 5, 10, 15);
printf("Product of 2, 3, 4, and 5: %d\n", p1);
printf("Product of 5, 10, and 15: %d\n", p2);
return 0;
}
Sample Output:
Product of 2, 3, 4, and 5: 120 Product of 5, 10, and 15: 750
Explanation:
In the above program, the multiply() function takes a variable number of arguments using the ... syntax. The function then initializes a 'va_list' variable args using the 'va_start()' macro and loops through each argument using the 'va_arg()' macro. The function multiplies each argument together to get the final product, which is then returned.
In the main() function, we call the multiply() function with a different number of arguments each time, and the function calculates the product of all the arguments passed to it.Flowchart:
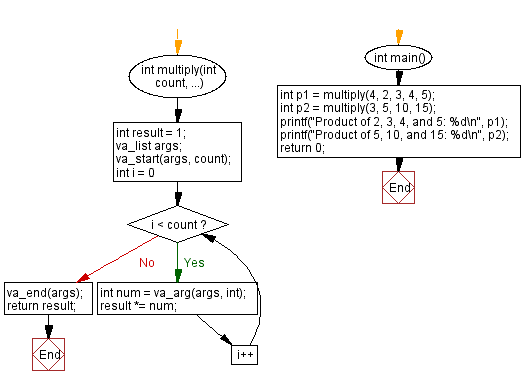
For more Practice: Solve these Related Problems:
- Write a C program to compute the product of a variable number of integers using a variadic function with a count parameter.
- Write a C program to implement a variadic product function that handles both positive and negative numbers correctly.
- Write a C program to recursively calculate the product of integers passed as variadic arguments and detect potential overflow.
- Write a C program to compute the product and also return the logarithm of the product for a variable number of integers using a variadic function.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Sum of a variable number of integers.
Next: Maximum and minimum of a variable number of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.