C Exercises: Sum of a variable number of integers
C Variadic function: Exercise-1 with Solution
Write a C program to find the sum of a variable number of integers passed as arguments to a function using variadic functions.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdarg.h>
int sum_nums(int ctr, ...) {
int sum_nums_all = 0;
va_list args;
va_start(args, ctr);
for (int i = 0; i < ctr; i++) {
int n = va_arg(args, int);
sum_nums_all += n;
}
va_end(args);
return sum_nums_all;
}
int main() {
int s1 = sum_nums(3, 1, 2, 3); // returns 6
int s2 = sum_nums(5, 1, 2, 3, 4, 5); // returns 15
int s3 = sum_nums(3, -1, -2, -3); // returns -6
printf("s1 = %d, s2 = %d, s3 = %d", s1, s2, s3);
return 0;
}
Sample Output:
s1 = 6, s2 = 15, s3 = -6
Explanation:
In the above program, the sum_nums() function takes two arguments, the number of integers to sum up and a variable number of arguments. It initializes a 'va_list' object args with the 'va_start' macro, and then iterates over the variable argument list using the 'va_arg' macro to retrieve each argument as an int. Finally, it cleans up the va_list object using the 'va_end' macro.
In the main function, we call the sum function thrice with different sets of integers, and print the results.
Flowchart:
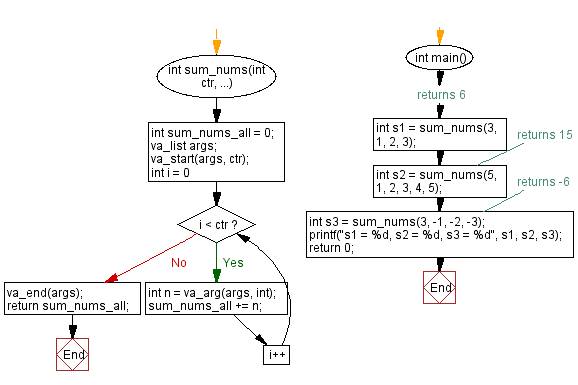
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: C Variadic function Exercises Home
Next: Product of a variable number of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics