C Program: Calculate Factorial with while loop and User-entered positive integer
6. Factorial Calculation Using a While Loop
Write a C program that prompts the user to enter a positive integer. It then calculates and prints the factorial of that number using a while loop.
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int number; // Variable to store the user input
long long factorial = 1; // Variable to store the factorial, initialized to 1
// Prompt the user to enter a positive integer
printf("Input a positive integer: ");
scanf("%d", &number); // Read the user input
// Check if the entered number is negative
if (number < 0) {
printf("Error: Input enter a non-negative integer.\n");
return 1; // Exit the program with an error code
}
// Calculate the factorial using a while loop
while (number > 0) {
factorial *= number; // Multiply the current factorial by the current number
number--; // Decrement the number for the next iteration
}
// Print the calculated factorial
printf("Factorial: %lld\n", factorial);
return 0; // Indicate successful program execution
}
Sample Output:
Input a positive integer: 5 Factorial: 120
Input a positive integer: -6 Error: Input enter a non-negative integer.
Explanation:
Here are key parts of the above code step by step:
- #include <stdio.h>: Include the standard input-output library for functions like "printf()" and "scanf()".
- int main() {: Start the main function.
- int number;: Declare an integer variable 'number' to store user input.
- long long factorial = 1;: Declare a long long variable 'factorial' and initialize it to 1 to store the factorial.
- printf("Enter a positive integer: ");: Prompt the user to enter a positive integer.
- scanf("%d", &number);: Read the user input and store it in the variable 'number'.
- if (number < 0) {: Check if the entered number is negative.
- printf("Error: Please input a non-negative integer.\n");: Print an error message if the number is negative.
- return 1;: Exit the program with an error code.
- while (number > 0) {: Start a while loop that continues as long as the 'number' is greater than 0.
- factorial *= number;: Multiply the current 'factorial' by the current 'number'.
- number--;: Decrement the 'number' for the next iteration.
- }: Close the while loop.
- printf("Factorial: %lld\n", factorial);: Print the calculated factorial using the %lld format specifier for long long.
- return 0;: Indicate successful program execution.
- }: Close the main function.
Flowchart:
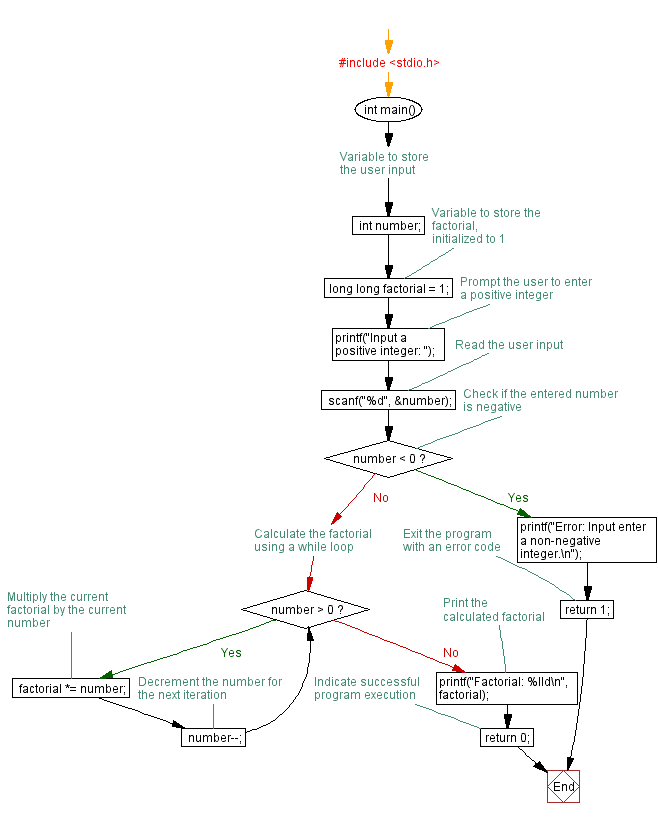
For more Practice: Solve these Related Problems:
- Write a C program to calculate the factorial of a positive integer using a while loop and then count the trailing zeros in the result.
- Write a C program to compute the factorial of a number using a while loop and determine whether the factorial is even or odd.
- Write a C program to calculate the factorial of a positive integer using a while loop and then compute the sum of the digits of the factorial.
- Write a C program to calculate the factorial using a while loop, but prompt the user for confirmation if the input number is greater than 10.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Guess the number game using while loop.
Next: C Program: User input validation with While Loop for minimum 8-Character username.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.