C Program: User input validation with While Loop for minimum 8-Character username
C While Loop: Exercise-7 with Solution
Write a C program that prompts the user to input a username. Use a while loop to keep asking for a username until a valid one is entered (e.g., at least 8 characters long).
Sample Solution:
C Code:
#include <stdio.h>
#include <string.h>
int main() {
char username[20]; // Array to store the user input for the username
// Start a while loop to keep asking for a valid username
while (1) {
// Prompt the user to input a username
printf("Input a username (at least 8 characters): ");
scanf("%s", username); // Read the user input and store it in the array
// Check if the entered username is at least 8 characters long
if (strlen(username) >= 8) {
break; // Exit the loop if the username is valid
} else {
// Print an error message if the username is too short
printf("Error: Username must be at least 8 characters long.\n");
}
// Clear the input buffer to handle any remaining characters
while (getchar() != '\n');
}
// Print a message indicating a valid username was entered
printf("Valid username entered: %s\n", username);
return 0; // Indicate successful program execution
}
Sample Output:
Input a username (at least 8 characters): Sdk34%k Error: Username must be at least 8 characters long. Input a username (at least 8 characters): Akdsui234#$ Valid username entered: Akdsui234#$
Explanation:
Here are key parts of the above code step by step:
- #include <stdio.h>: Include the standard input-output library for functions like "printf()" and "scanf()".
- #include <string.h>: Include the string manipulation library for functions like strlen.
- int main() {: Start the main function.
- char username[20];: Declare a character array 'username' to store the user input for the username.
- while (1) {: Start an infinite while loop.
- printf("Enter a username (at least 8 characters): ");: Prompt the user to enter a username.
- scanf("%s", username);: Read the user input and store it in the 'username' array.
- if (strlen(username) >= 8) {: Check if the entered username is at least 8 characters long using 'strlen'.
- break;: Exit the loop if the username is valid.
- } else { printf("Error: Username must be at least 8 characters long.\n"); }: Print an error message if the username is too short.
- while (getchar() != '\n');: Clear the input buffer to handle any remaining characters.
- }: Close the while loop.
- printf("Valid username entered: %s\n", username);: Print a message indicating a valid username was entered.
- return 0;: Indicate successful program execution.
- }: Close the main function.
Flowchart:
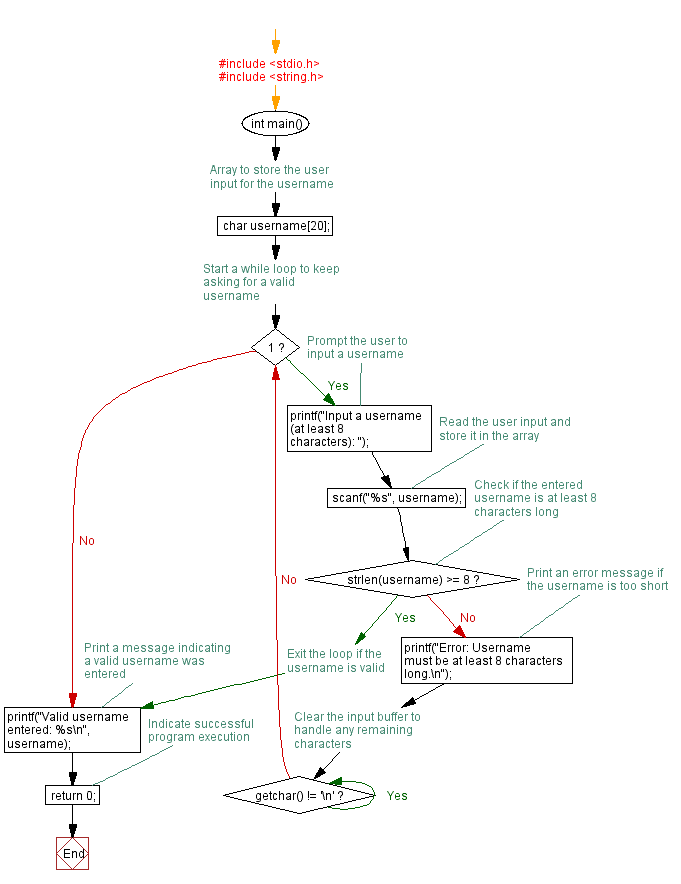
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Calculate Factorial with while loop and User-entered positive integer.
Next: Sum of Cubes of Even Numbers up to 20 using While loop
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics