C Program: Sum of Cubes of Even Numbers up to 20 using While loop
8. Sum of Cubes of Even Numbers Up to a Limit
Write a C program that calculates and prints the sum of cubes of even numbers up to a specified limit (e.g., 20) using a while loop.
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int limit = 20; // Specify the limit for even numbers
int num = 2; // Start with the first even number
int sum = 0; // Variable to store the sum of cubes
// Start a while loop to iterate through even numbers up to the limit
while (num <= limit) {
// Calculate the cube of the current even number
int cube = num * num * num;
// Add the cube to the sum
sum += cube;
// Move to the next even number
num += 2;
}
// Print the sum of cubes of even numbers
printf("Sum of cubes of even numbers up to %d: %d\n", limit, sum);
return 0; // Indicate successful program execution
}
Sample Output:
Sum of cubes of even numbers up to 20: 24200
Explanation:
Here are key parts of the above code step by step:
- #include <stdio.h>: Include the standard input-output library for functions like "printf()".
- int main() {: Start the main function.
- int limit = 20;: Specify the limit for even numbers.
- int num = 2;: Start with the first even number.
- int sum = 0;: Initialize a variable to store the sum of cubes.
- while (num <= limit) {: Start a while loop to iterate through even numbers up to the limit.
- int cube = num num num;: Calculate the cube of the current even number.
- sum += cube;: Add the cube to the sum.
- num += 2;: Move to the next even number.
- }: Close the while loop.
- printf("Sum of cubes of even numbers up to %d: %d\n", limit, sum);: Print the sum of cubes of even numbers.
- return 0;: Indicate successful program execution.
- }: Close the main function.
Flowchart:
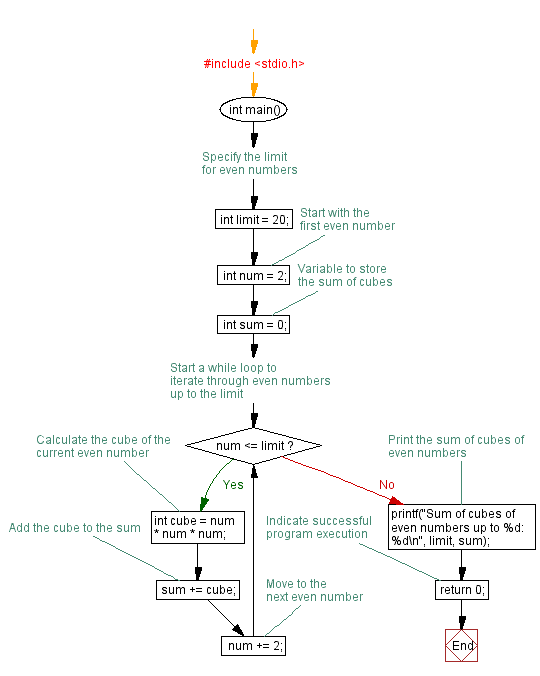
For more Practice: Solve these Related Problems:
- Write a C program to calculate the sum of cubes of even numbers up to a given limit using a while loop, skipping cubes divisible by 10.
- Write a C program to compute the sum of cubes of even numbers up to a specified limit and then subtract the sum of cubes of odd numbers.
- Write a C program to calculate the sum of cubes of even numbers up to a given limit using a while loop and then determine the average cube value.
- Write a C program to sum the cubes of even numbers up to a given limit using a while loop and output the result in scientific notation.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: User input validation with While Loop for minimum 8-Character username.
Next: Palindrome check using While loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.