C++ Exercises: Check if the sum of all 5' in the array exactly 15 in a given array of integers
C++ Basic Algorithm: Exercise-104 with Solution
Check if Sum of All '5's Equals 15
Write a C++ program that checks if the sum of all 5' in the array is exactly 15.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// Function definition that checks if the sum of 5s in the array equals 15
static bool test(int nums[], int arr_length)
{
int sum = 0; // Initializing variable to store the sum of 5s
// Loop through the array to count the occurrences of the number 5 and calculate the sum
for (int i = 0; i < arr_length; i++)
{
if (nums[i] == 5) // Checking if the element is equal to 5
sum += 5; // Incrementing sum by 5 if the element is 5
}
return sum == 15; // Returning true if the sum of 5s in the array equals 15, otherwise false
}
int main()
{
int nums1[] = {1, 5, 6, 9, 10, 17}; // Initializing array nums1 with various integers
int arr_length = sizeof(nums1) / sizeof(nums1[0]); // Calculating length of array nums1
// Calling test function with nums1 and displaying the result (true or false)
cout << test(nums1, arr_length) << endl;
int nums2[] = {1, 5, 5, 5, 10, 17}; // Initializing array nums2 with multiple occurrences of 5
arr_length = sizeof(nums2) / sizeof(nums2[0]); // Calculating length of array nums2
// Calling test function with nums2 and displaying the result (true or false)
cout << test(nums2, arr_length) << endl;
int nums3[] = { 1, 1, 5, 5, 5, 5 }; // Initializing array nums3 with consecutive occurrences of 5
arr_length = sizeof(nums3) / sizeof(nums3[0]); // Calculating length of array nums3
// Calling test function with nums3 and displaying the result (true or false)
cout << test(nums3, arr_length) << endl;
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
0 1 0
Visual Presentation:
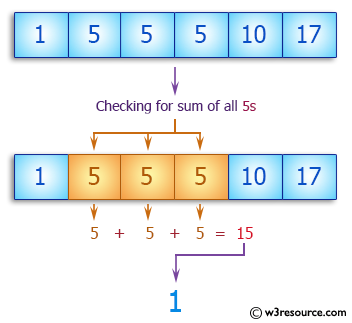
Flowchart:
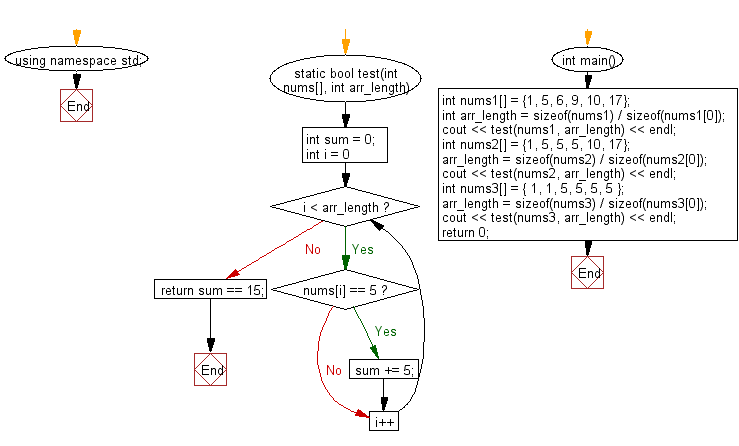
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check whether a given array of integers contains 5's and 7's.
Next: Write a C++ program to check if the number of 3's is greater than the number of 5's.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/basic-algorithm/cpp-basic-algorithm-exercise-104.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics