C++ Exercises: Check if the number of 3's is greater than the number of 5's
Compare Count of '3's and '5's in Array
Write a C++ program to check if the number of 3's is greater than the number of 5's.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// Function definition that compares the count of occurrences of 3 and 5 in an array
static bool test(int nums[], int arr_length)
{
int no_3 = 0, no_5 = 0; // Initializing variables to count occurrences of 3 and 5
// Loop through the array to count occurrences of 3 and 5
for (int i = 0; i < arr_length; i++)
{
if (nums[i] == 3) // Checking if the element is equal to 3
no_3++; // Incrementing count for 3 if the element is 3
if (nums[i] == 5) // Checking if the element is equal to 5
no_5++; // Incrementing count for 5 if the element is 5
}
return no_3 > no_5; // Returning true if occurrences of 3 are greater than occurrences of 5, otherwise false
}
int main()
{
int nums1[] = {1, 5, 6, 9, 3, 3}; // Initializing array nums1 with various integers including 3 and 5
int arr_length = sizeof(nums1) / sizeof(nums1[0]); // Calculating length of array nums1
// Calling test function with nums1 and displaying the result (true or false)
cout << test(nums1, arr_length) << endl;
int nums2[] = {1, 5, 5, 5, 10, 17}; // Initializing array nums2 with multiple occurrences of 5
arr_length = sizeof(nums2) / sizeof(nums2[0]); // Calculating length of array nums2
// Calling test function with nums2 and displaying the result (true or false)
cout << test(nums2, arr_length) << endl;
int nums3[] = {1, 3, 3, 5, 5, 5}; // Initializing array nums3 with multiple occurrences of 3 and 5
arr_length = sizeof(nums3) / sizeof(nums3[0]); // Calculating length of array nums3
// Calling test function with nums3 and displaying the result (true or false)
cout << test(nums3, arr_length) << endl;
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
1 0 0
Visual Presentation:
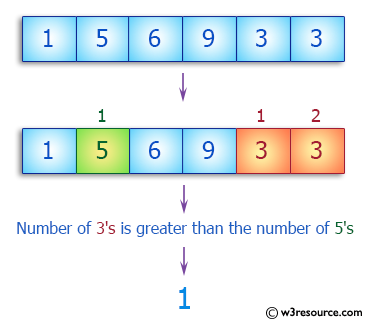
Flowchart:
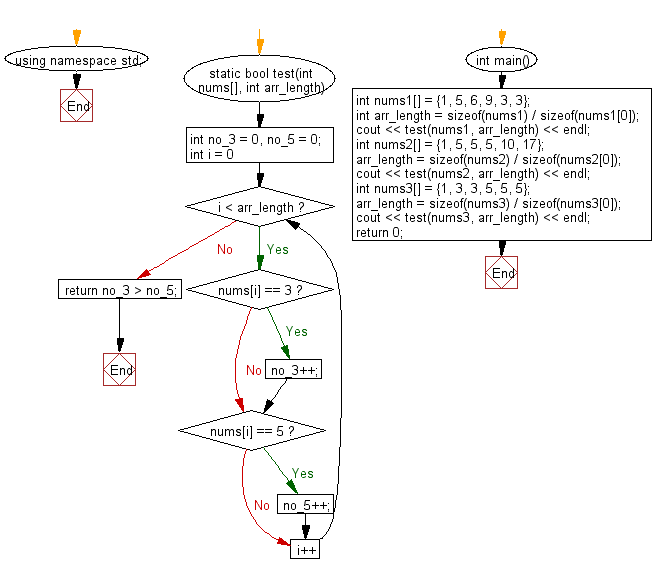
For more Practice: Solve these Related Problems:
- Write a C++ program to count the number of 3's and 5's in an array and return true if the count of 3's exceeds that of 5's.
- Write a C++ program that reads an array of integers and outputs a boolean result based on whether there are more 3's than 5's.
- Write a C++ program to compare the frequencies of 3 and 5 in an input array and print 1 if the count of 3's is greater.
- Write a C++ program that processes an integer array and returns true if the number of 3's is strictly larger than the number of 5's.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check if the sum of all 5' in the array exactly 15 in a given array of integers.
Next: Write a C++ program to check if a given array of integers contains a 3 or a 5.
What is the difficulty level of this exercise?