C++ Exercises: Check if a given array of integers contains a 3 or a 5
Check for Presence of '3' or '5' in Array
Write a C++ program to check if a given array of integers contains a 3 or a 5.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// Function definition that checks if all elements in the array are either 3 or 5
static bool test(int nums[], int arr_length)
{
// Loop through the array to check if all elements are either 3 or 5
for (int i = 0; i < arr_length; i++)
{
if (nums[i] != 3 && nums[i] != 5) // Checking if the element is neither 3 nor 5
return false; // Returning false if any element is different from 3 or 5
}
return true; // Returning true if all elements are either 3 or 5
}
int main()
{
int nums1[] = {5, 5, 5, 5, 5}; // Initializing array nums1 with all elements as 5
int arr_length = sizeof(nums1) / sizeof(nums1[0]); // Calculating length of array nums1
// Calling test function with nums1 and displaying the result (true or false)
cout << test(nums1, arr_length) << endl;
int nums2[] = {3, 3, 3, 3}; // Initializing array nums2 with all elements as 3
arr_length = sizeof(nums2) / sizeof(nums2[0]); // Calculating length of array nums2
// Calling test function with nums2 and displaying the result (true or false)
cout << test(nums2, arr_length) << endl;
int nums3[] = {3, 3, 3, 5, 5, 5}; // Initializing array nums3 with elements as both 3 and 5
arr_length = sizeof(nums3) / sizeof(nums3[0]); // Calculating length of array nums3
// Calling test function with nums3 and displaying the result (true or false)
cout << test(nums3, arr_length) << endl;
int nums4[] = {1, 6, 8, 10}; // Initializing array nums4 with elements other than 3 and 5
arr_length = sizeof(nums4) / sizeof(nums4[0]); // Calculating length of array nums4
// Calling test function with nums4 and displaying the result (true or false)
cout << test(nums4, arr_length) << endl;
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
1 1 1 0
Visual Presentation:
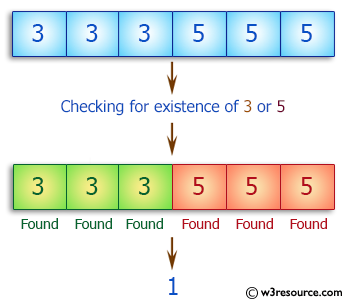
Flowchart:
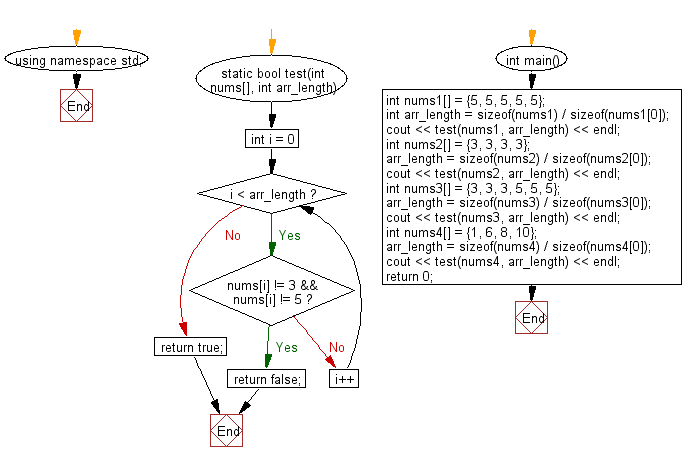
For more Practice: Solve these Related Problems:
- Write a C++ program to determine if an array contains at least one occurrence of either 3 or 5, returning true if found.
- Write a C++ program that reads an array of integers and outputs true if any element equals 3 or 5.
- Write a C++ program to scan an array and print 1 if it contains a 3 or a 5, and 0 otherwise.
- Write a C++ program that checks an input array for the presence of the digits 3 or 5, returning a boolean result.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check if the number of 3's is greater than the number of 5's.
Next: Write a C++ program to check if a given array of integers contains no 3 or a 5.
What is the difficulty level of this exercise?