C++ File handling: Sort text file lines alphabetically
8. Sort Lines in a Text File Alphabetically
Write a C++ program to sort the lines of a text file in alphabetical order.
Sample Solution:
C Code:
#include <iostream> // Including the input/output stream library
#include <fstream> // Including the file stream library
#include <vector> // Including the vector container
#include <algorithm> // Including algorithms like 'sort'
#include <iterator> // Including iterator operations
// Function to display the content of a file
void displayFileContent(const std::string & filename) {
std::ifstream file(filename); // Open file with given filename for reading
std::string line; // Declare a string to store each line of text
if (file.is_open()) { // Check if the file was successfully opened
std::cout << "File content:" << std::endl; // Displaying a message indicating file content
while (std::getline(file, line)) { // Read each line from the file
std::cout << line << std::endl; // Display each line of the file
}
file.close(); // Close the file
} else {
std::cout << "Failed to open the file." << std::endl; // Display an error message if file opening failed
}
}
int main() {
std::ifstream inputFile("test1.txt"); // Open the input file named "test1.txt" for reading
displayFileContent("test1.txt"); // Display content of "test1.txt"
std::ofstream outputFile("sorted_test1.txt"); // Create or overwrite the output file named "sorted_test1.txt" for writing
if (inputFile.is_open() && outputFile.is_open()) { // Check if both input and output files were successfully opened
std::vector<std::string> lines; // Vector to store the lines of the file
std::string line; // Declare a string variable to store each line of text
while (std::getline(inputFile, line)) { // Read each line from the input file and store it in the vector
lines.push_back(line); // Push each line to the vector
}
std::sort(lines.begin(), lines.end()); // Sort the lines in alphabetical order
std::copy(lines.begin(), lines.end(), std::ostream_iterator<std::string>(outputFile, "\n")); // Write the sorted lines to the output file
inputFile.close(); // Close the input file
outputFile.close(); // Close the output file
std::cout << "\nLines sorted successfully.\n" << std::endl; // Display a success message
displayFileContent("sorted_test1.txt"); // Display content of "sorted_test1.txt"
} else {
std::cout << "\nFailed to open the files." << std::endl; // Display an error message if file opening failed
}
return 0; // Return 0 to indicate successful execution
}
Sample Output:
File content: Many vendors provide C++ compilers, including the Free Software Foundation, LLVM, Microsoft, Intel, Embarcadero, Oracle, and IBM. C++ is a high-level, general-purpose programming language created by Danish computer scientist Bjarne Stroustrup. It is almost always implemented in a compiled language. Modern C++ currently has object-oriented, generic, and functional features, in addition to facilities for low-level memory manipulation. First released in 1985 as an extension of the C programming language, it has since expanded significantly over time. Lines sorted successfully. File content: C++ is a high-level, general-purpose programming language created by Danish computer scientist Bjarne Stroustrup. First released in 1985 as an extension of the C programming language, it has since expanded significantly over time. It is almost always implemented in a compiled language. Many vendors provide C++ compilers, including the Free Software Foundation, LLVM, Microsoft, Intel, Embarcadero, Oracle, and IBM. Modern C++ currently has object-oriented, generic, and functional features, in addition to facilities for low-level memory manipulation.
Explanation:
In the above exercise -
- Open the input file using std::ifstream and the output file using std::ofstream.
- Use a vector lines to store the input file lines. Read each line from the input file using std::getline() and push it into the vector.
- Use the std::sort() function from the <algorithm> library to sort the lines in alphabetical order.
- Write the sorted lines to the output file using std::copy() and std::ostream_iterator.
- After running the program, the input file lines will be sorted in alphabetical order and written to the output file.
Note: Content of a file is displayed using displayFileContent("filename.txt").
Flowchart:
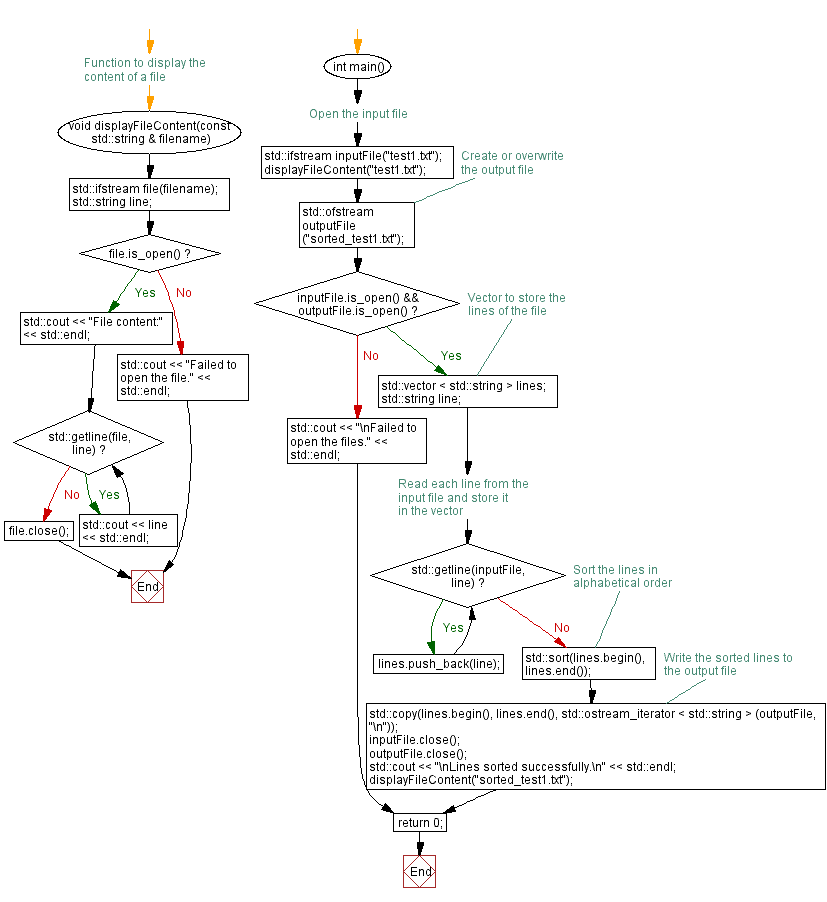
For more Practice: Solve these Related Problems:
- Write a C++ program to read all lines from a text file into a vector, sort the vector alphabetically, and output the sorted lines.
- Write a C++ program that opens a file, stores each line in an array, sorts the array using quicksort, and prints the sorted lines.
- Write a C++ program to dynamically read a file’s content into a list, sort the lines in alphabetical order, and write them back to the file.
- Write a C++ program to sort the lines of a text file by using the STL sort function and then display the ordered lines.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Append data to existing text file.
Next C++ Exercise: Merge multiple text files.
What is the difficulty level of this exercise?