C++ File handling: Merge multiple text files
9. Merge Multiple Text Files into One
Write a C++ program to merge multiple text files into a single file.
Sample Solution:
C Code:
#include <iostream> // Including the input/output stream library
#include <fstream> // Including the file stream library
#include <string> // Including the string handling library
#include <vector> // Including the vector container
// Function to display the content of a file
void displayFileContent(const std::string & filename) {
std::ifstream file(filename); // Open file with given filename for reading
std::string line; // Declare a string to store each line of text
if (file.is_open()) { // Check if the file was successfully opened
std::cout << "File content:" << std::endl; // Displaying a message indicating file content
while (std::getline(file, line)) { // Read each line from the file
std::cout << line << std::endl; // Display each line of the file
}
file.close(); // Close the file
} else {
std::cout << "Failed to open the file." << std::endl; // Display an error message if file opening failed
}
}
int main() {
std::vector<std::string> inputFiles = { // List of input files
"test1.txt",
"test2.txt",
"test3.txt",
"test4.txt"
};
std::cout << "Content of test1.txt, test2.txt, test3.txt, text4.txt: " << std::endl;
displayFileContent("test1.txt"); // Display content of "test1.txt"
displayFileContent("test2.txt"); // Display content of "test2.txt"
displayFileContent("test3.txt"); // Display content of "test3.txt"
displayFileContent("test4.txt"); // Display content of "test4.txt"
std::string outputFile = "merged_test_file.txt"; // Output file
std::ofstream mergedFile(outputFile); // Create or overwrite the output file named "merged_test_file.txt" for writing
if (mergedFile.is_open()) { // Check if the output file was successfully opened
for (const auto & inputFile: inputFiles) { // Iterate through each input file
std::ifstream inputFileStream(inputFile); // Open each input file for reading
if (inputFileStream.is_open()) { // Check if the input file was successfully opened
std::string line; // Declare a string to store each line of text
while (std::getline(inputFileStream, line)) { // Read each line from the input file
mergedFile << line << "\n"; // Write each line to the merged file
}
inputFileStream.close(); // Close the input file
} else {
std::cout << "Failed to open input file: " << inputFile << std::endl; // Display an error message if file opening failed
}
}
mergedFile.close(); // Close the merged file
std::cout << "\nFiles merged successfully." << std::endl; // Display a success message
std::cout << "\nContent of the merged file:" << std::endl;
displayFileContent("merged_test_file.txt"); // Display content of "merged_test_file.txt"
} else {
std::cout << "Failed to open the output file." << std::endl; // Display an error message if output file opening failed
}
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Content of test1.txt, test2.txt, test3.txt, text4.txt: File content: Many vendors provide C++ compilers, including the Free Software Foundation, LLVM, Microsoft, Intel, Embarcadero, Oracle, and IBM. File content: C++ is a high-level, general-purpose programming language created by Danish computer scientist Bjarne Stroustrup. File content: It is almost always implemented in a compiled language. File content: Modern C++ currently has object-oriented, generic, and functional features, in addition to facilities for low-level memory manipulation. First released in 1985 as an extension of the C programming language, it has since expanded significantly over time. Files merged successfully. Content of the merged file: File content: Many vendors provide C++ compilers, including the Free Software Foundation, LLVM, Microsoft, Intel, Embarcadero, Oracle, and IBM. C++ is a high-level, general-purpose programming language created by Danish computer scientist Bjarne Stroustrup. It is almost always implemented in a compiled language. Modern C++ currently has object-oriented, generic, and functional features, in addition to facilities for low-level memory manipulation. First released in 1985 as an extension of the C programming language, it has since expanded significantly over time.
Explanation:
In the above exercise,
- Define a vector inputFiles that contains the names of the input files to be merged.
- Specify the output file name as a string outputFile.
- The program uses an output file stream mergedFile to create or overwrite the output file.
- Iterate over each input file in the inputFiles vector.
- For each input file, open it using an input file stream inputFileStream.
- If the input file is successfully opened, read each line from the input file using std::getline() and write it to the merged file using the output file stream mergedFile.
- After merging all the files, close the merged file and display a success message.
Flowchart:
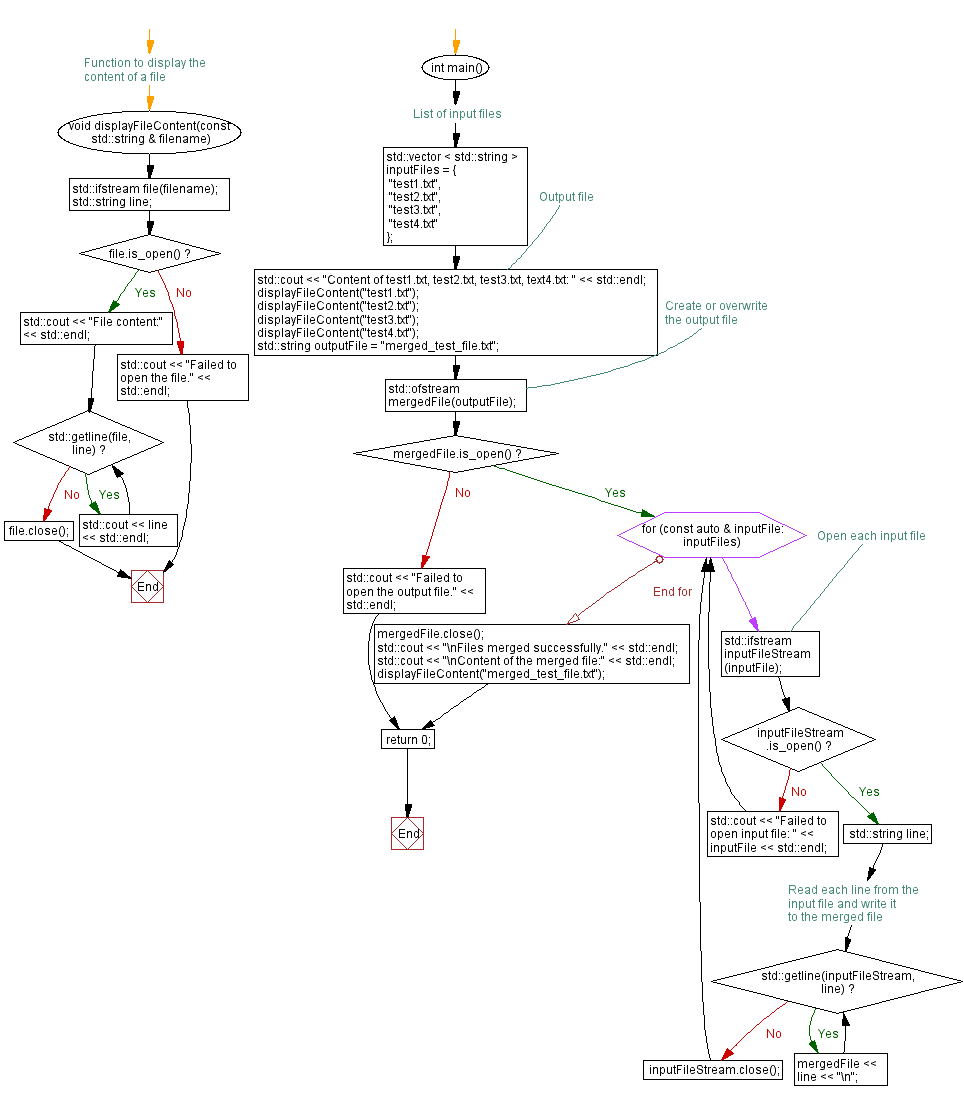
For more Practice: Solve these Related Problems:
- Write a C++ program to merge the contents of three text files into a single output file using file streams.
- Write a C++ program that reads from multiple files sequentially and writes their combined contents to a new file.
- Write a C++ program to dynamically merge text files provided by the user and display the merged content on the console.
- Write a C++ program to create a master file by concatenating several text files, ensuring that the files are merged in alphabetical order.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Sort text file lines alphabetically.
Next C++ Exercise: Split a large text file.
What is the difficulty level of this exercise?