C++ Exercises: Find the frequency of each digit in a given integer
59. Frequency of Each Digit in an Integer
Write a program in C++ to find the frequency of each digit in a given integer.
Visual Presentation:
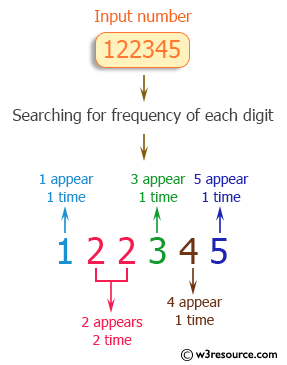
Sample Solution:-
C++ Code :
#include <iostream> // Include the input/output stream library
using namespace std; // Using standard namespace
int main() // Main function where the execution of the program starts
{
int n, i, j, ctr, r; // Declare integer variables n, i, j, ctr, and r
// Display message asking for input
cout << "\n\n Find frequency of each digit in a given integer:\n";
cout << "-----------------------------------------------------\n";
cout << " Input any number: ";
cin >> n; // Read input for the number from the user
// Loop through each digit from 0 to 9 to find their frequency
for (i = 0; i < 10; i++)
{
cout << "The frequency of " << i << " = "; // Display the current digit
ctr = 0; // Initialize the counter for the current digit's frequency
// Loop through the number to count occurrences of the current digit
for (j = n; j < 0; j = j / 10)
{
r = j % 10; // Extract the last digit of the number
if (r == i) // Check if the extracted digit is equal to the current digit being checked
{
ctr++; // Increment the counter for the current digit's frequency
}
}
// Display the frequency of the current digit
cout << ctr << endl;
}
}
Sample Output:
Find frequency of each digit in a given integer: ----------------------------------------------------- Input any number: 122345 The frequency of 0 = 0 The frequency of 1 = 1 The frequency of 2 = 2 The frequency of 3 = 1 The frequency of 4 = 1 The frequency of 5 = 1 The frequency of 6 = 0 The frequency of 7 = 0 The frequency of 8 = 0 The frequency of 9 = 0
Flowchart:
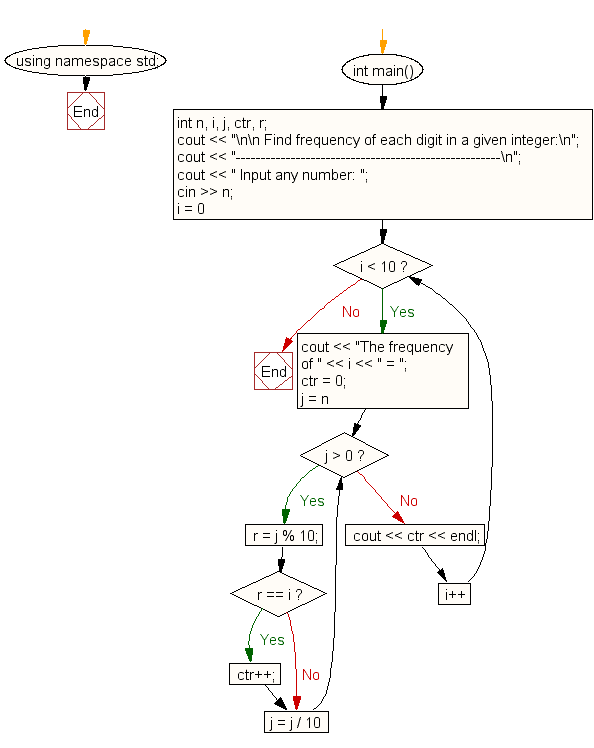
For more Practice: Solve these Related Problems:
- Write a C++ program to count and display the frequency of each digit (0-9) in a given integer.
- Write a C++ program that reads an integer, converts it to a string, and uses an array to track the count of each digit.
- Write a C++ program to iterate through the digits of a number and print the frequency for each digit from 0 to 9.
- Write a C++ program that processes an input number and outputs a formatted report of how many times each digit appears.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to calculate product of digits of any number.
Next: Write a program in C++ to input any number and print it in words.
What is the difficulty level of this exercise?