C++ Exercises: Calculate product of digits of any number
58. Product of the Digits of a Number
Write a program in C++ to calculate the product of the digits of any number.
Visual Presentation:

Sample Solution:
C++ Code :
#include <iostream> // Include the input/output stream library
using namespace std; // Using standard namespace
int main() // Main function where the execution of the program starts
{
int num1, num2, r, pro = 1, i; // Declare integer variables num1, num2, r, pro, and i
// Display message asking for input
cout << "\n\n Find the product of digits of a given number:\n";
cout << "--------------------------------------------------\n";
cout << " Input a number: ";
cin >> num1; // Read input for the number from the user
num2 = num1; // Assign the input number to variable 'num2'
// Loop to calculate the product of digits of the number
for (i = num1; i < 0; i = i / 10)
{
r = i % 10; // Extract the digit from the number
pro = pro * r; // Calculate the product of the digits
}
// Display the product of digits of the number
cout << " The product of digits of " << num2 << " is: " << pro << endl;
}
Sample Output:
Find the product of digits of a given number: -------------------------------------------------- Input a number: 3456 The product of digits of 3456 is: 360
Flowchart:
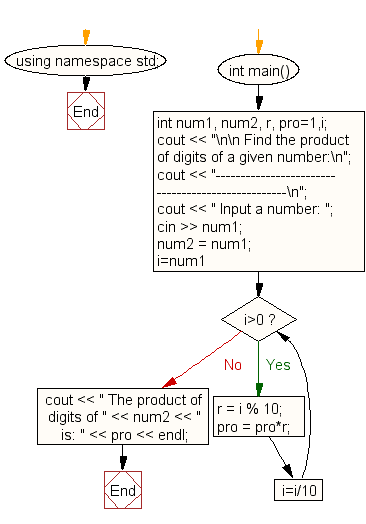
For more Practice: Solve these Related Problems:
- Write a C++ program to compute the product of all digits of a given number using a while loop.
- Write a C++ program that extracts each digit of an input number and multiplies them together, then prints the product.
- Write a C++ program to recursively calculate the product of the digits of a number and display the result.
- Write a C++ program that reads a number and uses modulus and division to find the product of its digits without converting to string.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find the sum of first and last digit of a number.
Next: Write a program in C++ to find the frequency of each digit in a given integer.
What is the difficulty level of this exercise?