C++ Exercises: Convert a binary number to octal number
C++ For Loop: Exercise-75 with Solution
Write a C++ program to convert a binary number to an octal number.
Visual Presentation:
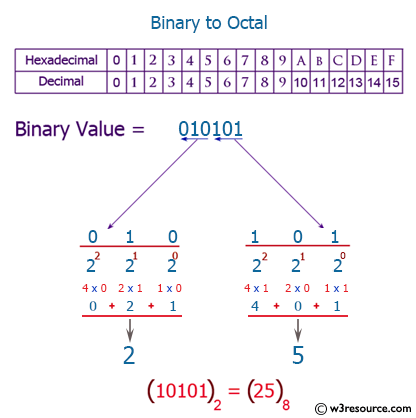
Sample Solution:-
C++ Code :
#include <iostream> // Include input/output stream library
#include <math.h> // Include math library for mathematical functions
using namespace std; // Use the standard namespace
int main() // Main function where the execution of the program starts
{
int binnum1, binaryNumber, rem, decnum = 0, quot, i = 1, j; // Declare variables for binary, decimal, quotient, and iteration
int octnum[100]; // Declare an array to store octal digits
cout << "\n\n Convert a binary number to octal number:\n"; // Display message prompting for binary to octal conversion
cout << "---------------------------------------------\n"; // Display separator line
cout << " Input a binary number: "; // Prompt user to input a binary number
cin >> binaryNumber; // Read the binary number input by the user
binnum1 = binaryNumber; // Store the input binary number for display later
while (binaryNumber > 0) // Loop to convert binary to decimal
{
rem = binaryNumber % 10; // Calculate the remainder by performing modulo 10 operation
decnum = decnum + rem * i; // Calculate the decimal number by adding the remainder multiplied by a factor 'i'
i = i * 2; // Multiply the factor 'i' by 2 for the next iteration
binaryNumber = binaryNumber / 10; // Update the binary number by removing its last digit (rightmost digit)
}
i = 1; // Reset the iteration variable to 1
quot = decnum; // Store the decimal number in 'quot'
while (quot > 0) // Loop to convert decimal to octal
{
octnum[i++] = quot % 8; // Calculate the remainder after dividing by 8 and store it in the octal array
quot = quot / 8; // Update the quotient by dividing by 8
}
cout << " The equivalent octal value of " << binnum1 << " is : "; // Display the equivalent octal value
for (j = i - 1; j > 0; j--) // Loop to display the octal digits
{
cout << octnum[j]; // Display octal digits in reverse order
}
cout << "\n"; // Output newline for formatting
}
Sample Output:
Convert a binary number to octal number: --------------------------------------------- Input a binary number: 1011 The equivalent octal value of 1011 is : 13
Flowchart:
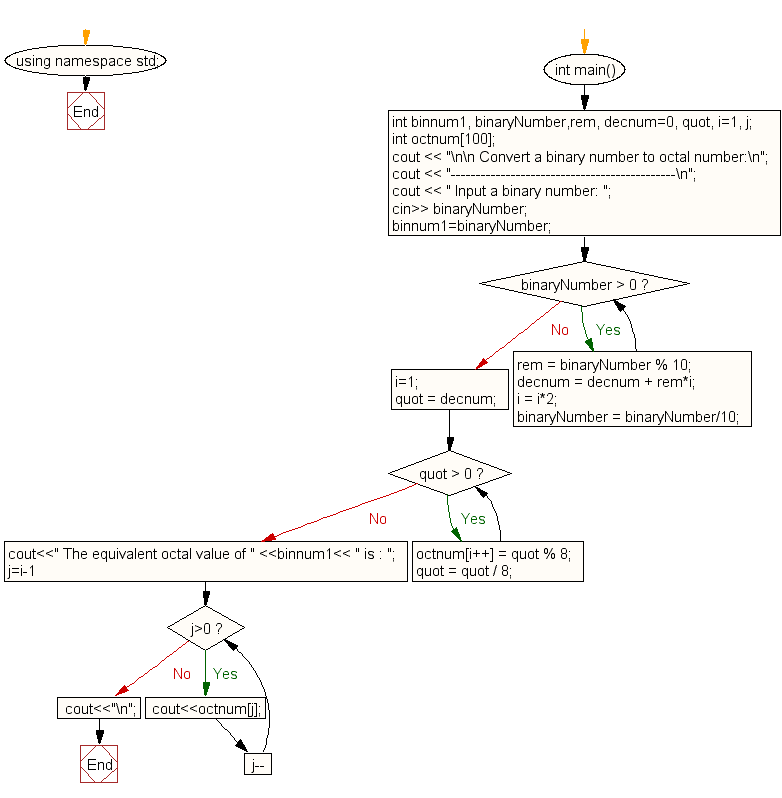
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to convert a binary number to hexadecimal number.
Next: Write a program in C++ to convert a octal number to decimal number.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics