C++ Linked List Exercises: Create and display linked list
1. Create and Display Singly Linked List
Write a C++ program to create and display a Singly Linked List.
Visualization:
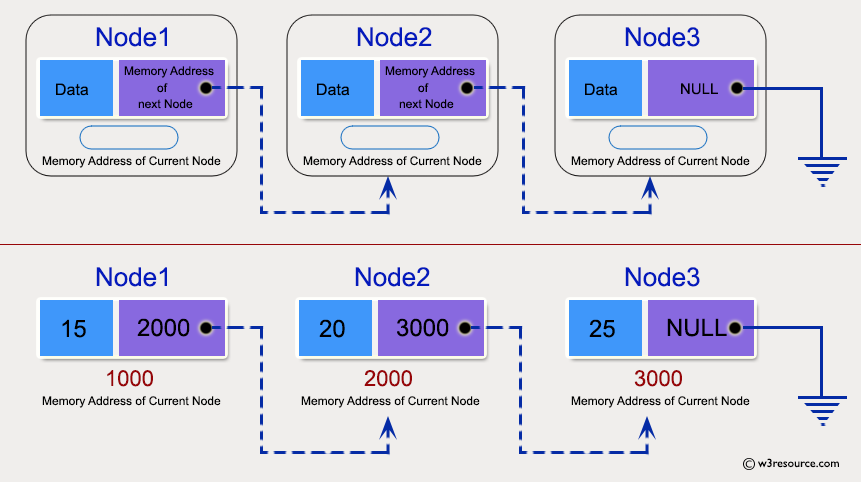
Test Data:
The list contains the data entered:
11 9 7 5 3 1
Sample Solution:
C++ Code:
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// Declare node structure
struct Node{
int num;
Node *next;
};
// Starting (Head) node
struct Node *head=NULL;
// Function to insert node at the start
void insert_Node(int n){
struct Node *new_node=new Node; // Creating a new node
new_node->num=n; // Assigning data to the new node
new_node->next=head; // Pointing the new node to the current head
head=new_node; // Making the new node as the head
}
// Function to display all nodes
void display_all_nodes(){
cout<<"The list contains the data entered:\n"; // Displaying a message
struct Node *temp = head; // Temporary node to traverse the list
while(temp!=NULL){ // Loop to iterate through the list
cout<<temp->num<<" "; // Displaying the data in the current node
temp=temp->next; // Moving to the next node
}
cout<<endl; // Displaying newline after printing all nodes
}
int main(){
insert_Node(1); // Inserting a node with value 1
insert_Node(3); // Inserting a node with value 3
insert_Node(5); // Inserting a node with value 5
insert_Node(7); // Inserting a node with value 7
insert_Node(9); // Inserting a node with value 9
insert_Node(11); // Inserting a node with value 11
display_all_nodes(); // Displaying all nodes in the list
return 0; // Returning from the main function
}
Sample Output:
The list contains the data entered: 11 9 7 5 3 1
Flowchart:
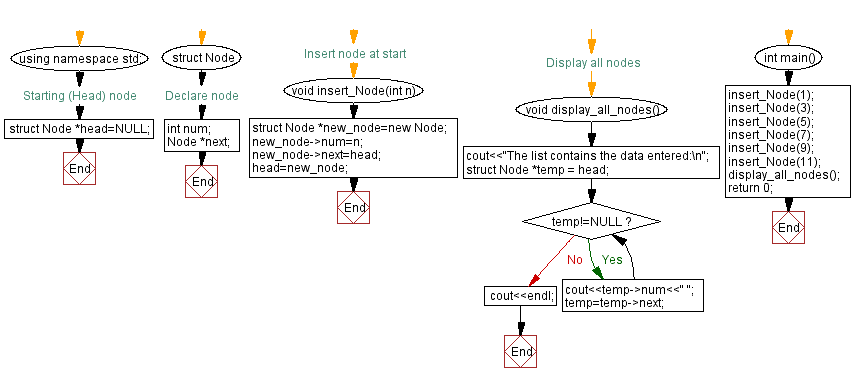
For more Practice: Solve these Related Problems:
- Develop a C++ program to build a singly linked list from user input and display the list using iterative traversal.
- Write a C++ program that creates a singly linked list with predefined values and prints each node along with its memory address.
- Design a C++ program to construct a singly linked list and display the list in a tabular format, marking the head and tail distinctly.
- Implement a C++ program to create a singly linked list using dynamic memory allocation and then display its elements with pointer arithmetic.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: C++ Linked List Exercises Home
Next C++ Exercise: Reverse linked list.
What is the difficulty level of this exercise?