C++ Linked List Exercises: Reverse linked list
C++ Linked List: Exercise-2 with Solution
Write a C++ program to create a singly linked list of n nodes and display it in reverse order.
Visualization:
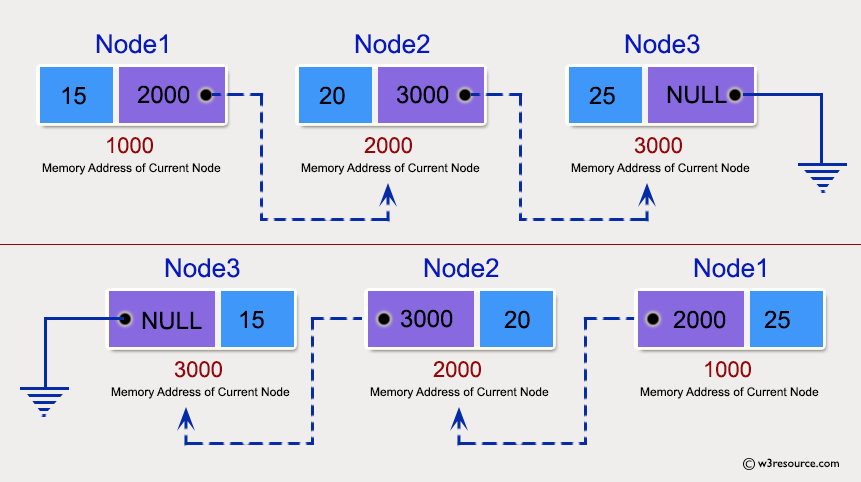
Test Data:
Original Linked list:
11 9 7 5 3 1
Reverse Linked list:
1 3 5 7 9 11
Sample Solution:
C++ Code:
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
//Declare node
struct node{
int num; // Data field to store a number
node *next; // Pointer to the next node
};
//Starting (Head) node
struct node *head=NULL; // Initializing the head node as NULL initially
//Insert node at start
void insert_Node(int n){
struct node *new_node=new node; // Creating a new node
new_node->num=n; // Assigning data to the new node
new_node->next=head; // Pointing the new node to the current head
head=new_node; // Making the new node as the head
}
// Function to reverse the linked list
void reverse() {
// Set the current, previous, and next pointers to their initial values
node* current = head;
node *prev = NULL, *next = NULL;
while (current != NULL) {
// Store next
next = current->next;
// Reverse the node pointer for the current node
current->next = prev;
// Advance the pointer one position.
prev = current;
current = next;
}
head = prev; // Make the last node (prev) as the new head of the reversed list
}
//Display all nodes
void display_all_nodes() {
struct node *temp = head; // Temporary node to traverse the list
while(temp != NULL) { // Loop to iterate through the list
cout << temp->num << " "; // Displaying the data in the current node
temp = temp->next; // Moving to the next node
}
cout << endl; // Displaying newline after printing all nodes
}
int main(){
insert_Node(1); // Inserting a node with value 1
insert_Node(3); // Inserting a node with value 3
insert_Node(5); // Inserting a node with value 5
insert_Node(7); // Inserting a node with value 7
insert_Node(9); // Inserting a node with value 9
insert_Node(11); // Inserting a node with value 11
cout << "Original Linked list:\n";
display_all_nodes(); // Displaying all nodes in the original list
cout << "\nReverse Linked list:\n";
reverse(); // Reversing the linked list
display_all_nodes(); // Displaying all nodes in the reversed list
return 0; // Returning from the main function
}
Sample Output:
Original Linked list: 11 9 7 5 3 1 Reverse Linked list: 1 3 5 7 9 11
Flowchart:
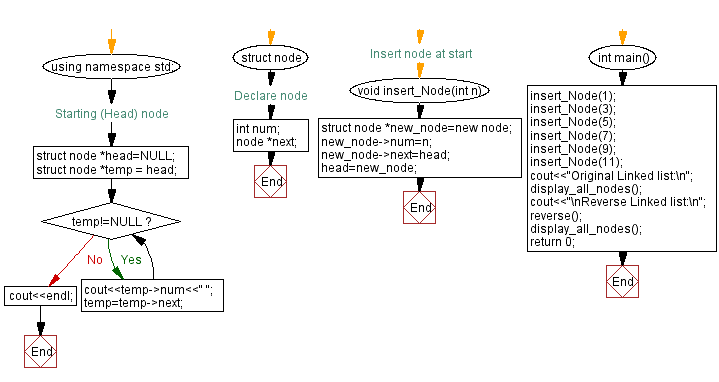
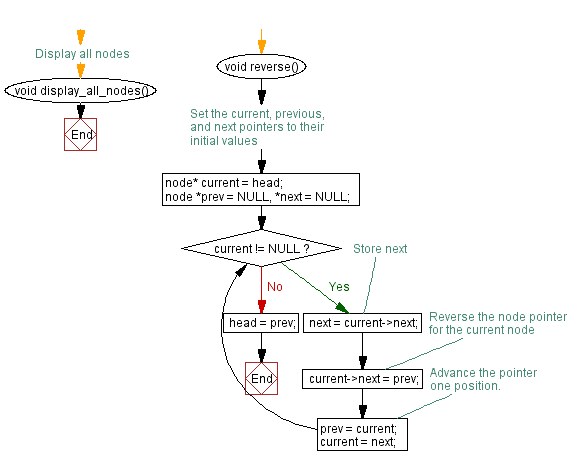
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Create and display linked list.
Next C++ Exercise: Count number of nodes in a linked list.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/linked_list/cpp-linked-list-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics