C++ Heapsort sort algorithm Exercise: Sort an array of elements using the Heapsort sort algorithm
8. Sort an Array Using the Heapsort Algorithm
Write a C++ program to sort an array of elements using the Heapsort sort algorithm.
Sample Solution:
C++ Code :
// Including necessary C++ libraries
#include <algorithm>
#include <iterator>
#include <iostream>
// Function to perform heap sort algorithm
template<typename RandomAccessIterator>
void heap_sort(RandomAccessIterator begin, RandomAccessIterator end) {
// Creating a heap from the elements in the range [begin, end)
std::make_heap(begin, end);
// Sorting the heap by repeatedly extracting the maximum element
std::sort_heap(begin, end);
}
// Main function
int main() {
// Initializing an array of integers for sorting
int a[] = {125, 0, 695, 3, -256, -5, 214, 44, 55};
// Displaying the original numbers in the array
std::cout << "Original numbers:\n";
std::copy(std::begin(a), std::end(a), std::ostream_iterator<int>(std::cout, " "));
std::cout << "\n";
// Sorting the array using heap_sort function
heap_sort(std::begin(a), std::end(a));
// Displaying the sorted numbers after heap sort
std::cout << "Sorted numbers:\n";
std::copy(std::begin(a), std::end(a), std::ostream_iterator<int>(std::cout, " "));
std::cout << "\n";
// Returning 0 to indicate successful execution
return 0;
}
Sample Output:
Original numbers: 125 0 695 3 -256 -5 214 44 55 Sorted numbers: -256 -5 0 3 44 55 125 214 695
Flowchart:
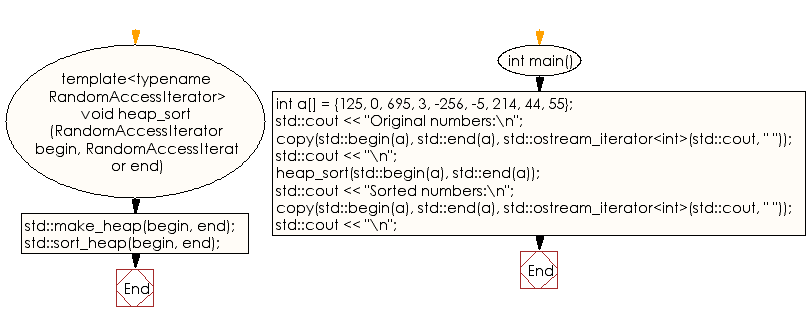
For more Practice: Solve these Related Problems:
- Write a C++ program to implement heapsort and print the heap structure after each extraction.
- Write a C++ program to implement heapsort with a custom comparator to sort in descending order.
- Write a C++ program to build both max-heap and min-heap versions of heapsort and compare their outputs.
- Write a C++ program to validate heapsort by comparing its output with STL's sort function.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to sort an array of elements using the Gnome sort algorithm.
Next: Write a C++ program to sort an array of elements using the Insertion sort algorithm.
What is the difficulty level of this exercise?