C++ Insertion sort algorithm Exercise: Sort an array of elements using the Insertion sort algorithm
9. Sort an Array Using the Insertion Sort Algorithm
Write a C++ program to sort an array of elements using the Insertion sort algorithm.
Sample Solution:
C++ Code :
// Including necessary C++ libraries
#include <algorithm>
#include <iostream>
#include <iterator>
// Function to perform insertion sort algorithm with a custom predicate
template <typename RandomAccessIterator, typename Predicate>
void insertion_sort(RandomAccessIterator begin, RandomAccessIterator end,
Predicate p) {
// Iterate through each element in the range [begin, end)
for (auto i = begin; i != end; ++i) {
// Rotate the sorted part to insert the current element at its correct position
std::rotate(std::upper_bound(begin, i, *i, p), i, i + 1);
}
}
// Function to perform insertion sort algorithm using the default 'less' comparison
template <typename RandomAccessIterator>
void insertion_sort(RandomAccessIterator begin, RandomAccessIterator end) {
// Utilizing insertion_sort function with 'less' as the default comparison
insertion_sort(
begin, end,
std::less<
typename std::iterator_traits<RandomAccessIterator>::value_type>());
}
// Main function
int main() {
// Initializing an array of integers for sorting
int a[] = {125, 0, 695, 3, -256, -5, 214, 44, 55};
// Displaying the original numbers in the array
std::cout << "Original numbers:\n";
std::copy(std::begin(a), std::end(a), std::ostream_iterator<int>(std::cout, " "));
std::cout << "\n";
// Sorting the array using insertion_sort function
insertion_sort(std::begin(a), std::end(a));
// Displaying the sorted numbers after insertion sort
std::cout << "Sorted numbers:\n";
std::copy(std::begin(a), std::end(a), std::ostream_iterator<int>(std::cout, " "));
std::cout << "\n";
// Returning 0 to indicate successful execution
return 0;
}
Sample Output:
Original numbers: 125 0 695 3 -256 -5 214 44 55 Sorted numbers: -256 -5 0 3 44 55 125 214 695
Flowchart:
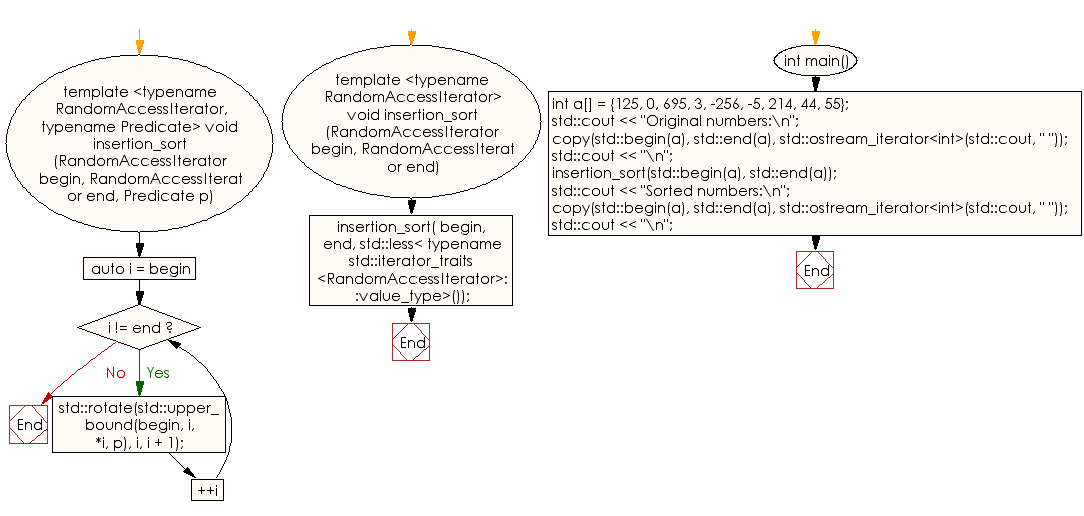
For more Practice: Solve these Related Problems:
- Write a C++ program to implement insertion sort and count the number of shifts for each element insertion.
- Write a C++ program to implement insertion sort recursively and display the array after each insertion.
- Write a C++ program to implement insertion sort with binary search to find the correct insertion position.
- Write a C++ program to sort an array of custom objects using insertion sort and overloaded comparison operators.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to sort an array of elements using the Heapsort sort algorithm.
Next: Write a C++ program to sort a collection of integers using the Merge sort.
What is the difficulty level of this exercise?