C#: Count the number of even elements in a given array of integers
Count Even Elements in Array
Write a C# Sharp program to count the even number of elements in a given array of integers.
Visual Presentation:
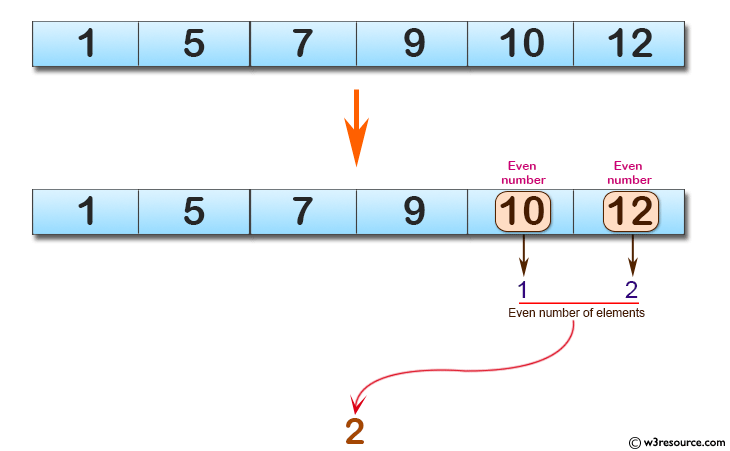
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Outputting the result of the 'test' method with an integer array as an argument
Console.WriteLine(test(new[] { 1, 5, 7, 9, 10, 12 }));
}
// Method to count the number of even integers in the given array
static int test(int[] nums)
{
int evens = 0; // Initializing a counter to keep track of the even numbers
// Looping through each element of the array
for (int i = 0; i < nums.Length; i++)
{
// Checking if the current element is even (divisible by 2)
if (nums[i] % 2 == 0)
{
evens++; // Incrementing the counter if the element is even
}
}
return evens; // Returning the total count of even numbers in the array
}
}
}
Sample Output:
2
Flowchart:
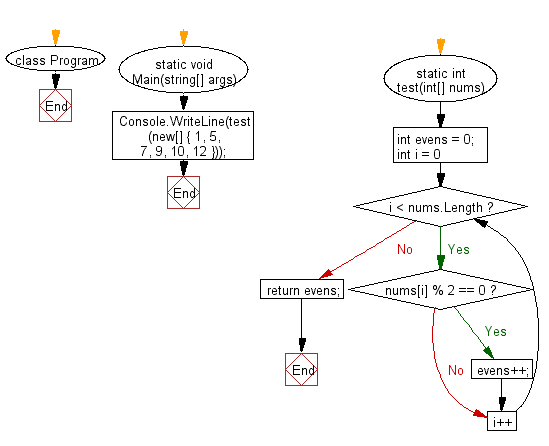
Go to:
PREV :First Two Elements or Full Array.
NEXT : Difference Between Largest and Smallest Values.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.