C#: Create a new array taking the first two elements from a given array
C# Sharp Basic Algorithm: Exercise-108 with Solution
Write a C# Sharp program to create an array by taking the first two elements from an array. If the given array length is shorter than 2, return the given array.
Visual Presentation:
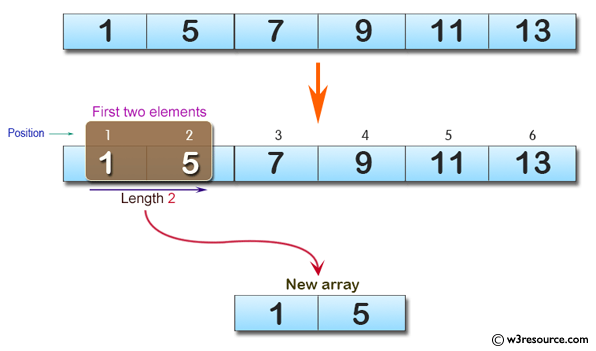
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Testing the 'test' method with an integer array as an argument and displaying the resulting array
int[] item = test(new[] { 1, 5, 7, 9, 11, 13 });
// Outputting text to indicate the start of displaying the new array
Console.Write("New array: ");
// Looping through each element of the 'item' array and displaying them
foreach(var i in item)
{
Console.Write(i.ToString()+" "); // Displaying each element of the 'item' array separated by a space
}
}
// Method to create and return a new array containing the first one or two elements of the input array
static int[] test(int[] numbers)
{
int[] front_nums; // Declaring an array to store the first one or two elements
// Checking the length of the input array to determine the elements to be included in the new array
if (numbers.Length >= 2)
{
front_nums = new int[] { numbers[0], numbers[1] }; // Creating an array with the first two elements
}
else if (numbers.Length > 0)
{
front_nums = new int[] { numbers[0] }; // Creating an array with the only available element
}
else
{
front_nums = new int[0]; // Creating an empty array if there are no elements in the input array
}
return front_nums; // Returning the new array containing the selected elements
}
}
}
Sample Output:
New array: 1 5
Flowchart:
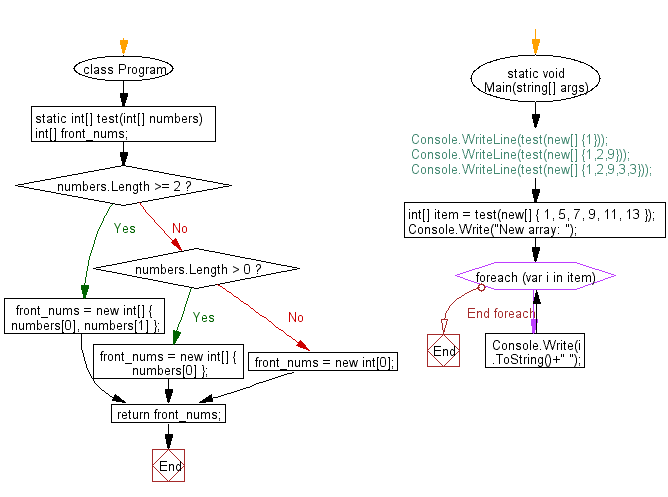
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to find the largest value from first, last, and middle elements of a given array of integers of odd length (atleast 1).
Next: Write a C# Sharp program to count even number of elements in a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics