C#: Find the largest value from first, last, and middle elements of a given array of integers of odd length (at least 1)
Largest Among First, Middle, and Last Elements
Write a C# Sharp program to find the largest value from first, last, and middle elements of a given array of integers of odd length (at least 1).
Visual Presentation:
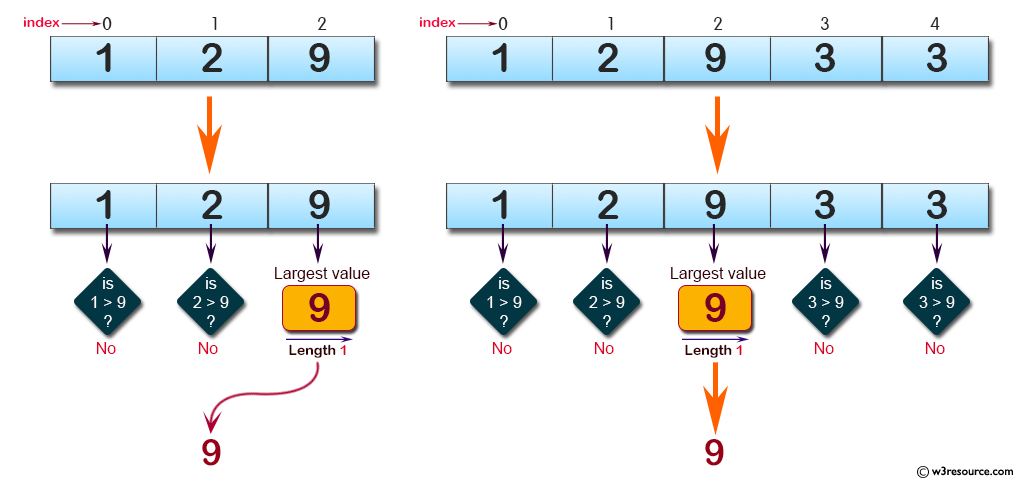
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Outputting the result of the 'test' method with various integer arrays as arguments
Console.WriteLine(test(new[] {1}));
Console.WriteLine(test(new[] {1,2,9}));
Console.WriteLine(test(new[] {1,2,9,3,3}));
Console.WriteLine(test(new[] {1,2,3,4,5,6,7}));
Console.WriteLine(test(new[] {1,2,2,3,7,8,9,10,6,5,4}));
}
// Method to find and return the maximum value among the first, middle, and last elements of an array
static int test(int[] numbers)
{
// Variables to store the first, middle, last, and maximum elements of the input array
int first, middle_ele, last_ele, max_ele;
// Storing the values of the first, middle, and last elements in respective variables
first = numbers[0];
middle_ele = numbers[numbers.Length / 2];
last_ele = numbers[numbers.Length - 1];
max_ele = first; // Assuming the maximum element is initially the first element
// Checking if the middle element is greater than the current maximum element
if (middle_ele > max_ele)
{
max_ele = middle_ele; // Updating the maximum element if the middle element is larger
}
// Checking if the last element is greater than the current maximum element
if (last_ele > max_ele)
{
max_ele = last_ele; // Updating the maximum element if the last element is larger
}
return max_ele; // Returning the maximum element found among first, middle, and last elements
}
}
}
Sample Output:
1 9 9 7 8
Flowchart:
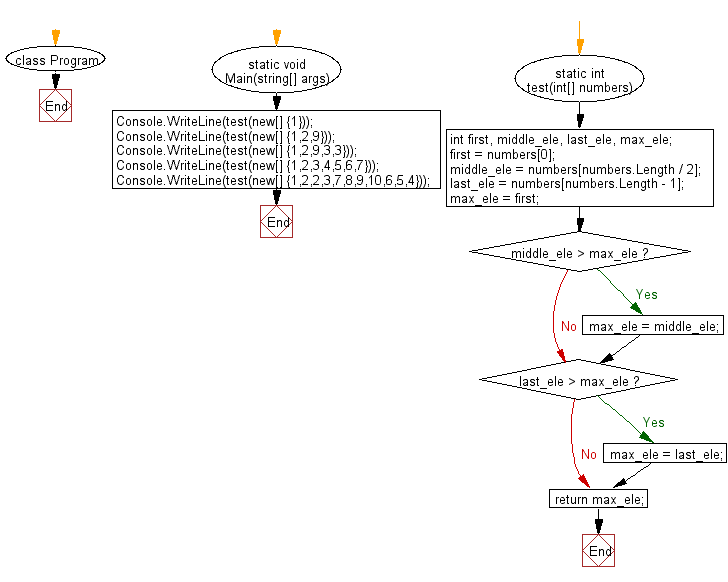
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new array length 4 from a given array (length atleast 4) containing the elements from the middle of the array.
Next: Write a C# Sharp program to create a new array taking the first two elements from a given array. If the length of the given array is less than 2 then return the give array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.