C#: Calculate the sum of the series [ 1-X^2/2!+X^4/4!- .........]
C# Sharp For Loop: Exercise-18 with Solution
Write a program in C# Sharp to find the sum of the series [ 1-X^2/2!+X^4/4!- .........].
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise18 // Declaration of the Exercise18 class
{
public static void Main() // Main method, entry point of the program
{
double x, sum, t, d; // Declaration of variables of type double
int i, n; // Declaration of variables of type integer
Console.Write("\n\n"); // Displaying new lines
Console.Write("Calculate the sum of the series [ 1-X^2/2!+X^4/4!- .........]:\n"); // Displaying the purpose of the program
Console.Write("----------------------------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input the Value of x :"); // Prompting the user to input the value of x
x = Convert.ToInt32(Console.ReadLine()); // Reading the value of x entered by the user
Console.Write("Input the number of terms : "); // Prompting the user to input the number of terms
n = Convert.ToInt32(Console.ReadLine()); // Reading the number of terms entered by the user
sum = 1; // Initializing the sum to 1 (as the series starts with 1)
t = 1; // Initializing t to 1 (initial term)
// Loop to calculate the sum of the series
for (i = 1; i < n; i++)
{
d = (2 * i) * (2 * i - 1); // Calculation of denominator value (factorial)
t = -t * x * x / d; // Calculation of the current term in the series
sum = sum + t; // Adding the current term to the sum
}
// Displaying the calculated sum, number of terms, and value of x
Console.Write("\nThe sum = {0}\nNumber of terms = {1}\nValue of x = {2}\n", sum, n, x);
}
}
Sample Output:
Calculate the sum of the series [ 1-X^2/2!+X^4/4!- .........]: ---------------------------------------------------------------- Input the Value of x :5 Input the number of terms : 2 the sum = -11.5 Number of terms = 2 value of x = 5
Flowchart:
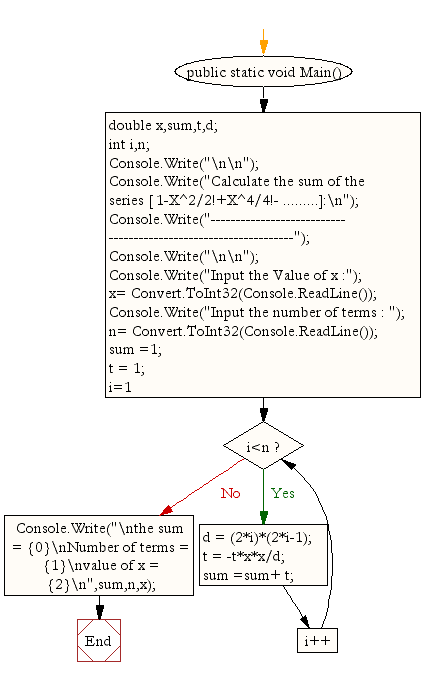
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to make such a pattern like a pyramid with number which will repeat the number in the same row.
Next: Write a program in C# Sharp to calculate the harmonic series and their sum.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/for-loop/csharp-for-loop-exercise-18.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics