C#: Display the pattern like a pyramid with a number which will repeat the number in the same row
C# Sharp For Loop: Exercise-17 with Solution
Write a program in C# Sharp to make such a pattern like a pyramid with a number which will repeat the number in the same row.
The pattern is as follows:
1 2 2 3 3 3 4 4 4 4
Visual Presentation:
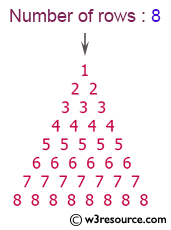
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise17 // Declaration of the Exercise17 class
{
public static void Main() // Main method, entry point of the program
{
int i, j, spc, rows, k; // Declaration of variables
Console.Write("\n\n"); // Displaying new lines
Console.Write("Display the pattern like pyramid with repeating a number in the same row:\n"); // Displaying the purpose of the program
Console.Write("-----------------------------------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input number of rows : "); // Prompting the user to input the number of rows
rows = Convert.ToInt32(Console.ReadLine()); // Reading the number of rows entered by the user
spc = rows + 4 - 1; // Setting up spaces for the pattern
// Loop to create the pattern
for (i = 1; i <= rows; i++)
{
for (k = spc; k >= 1; k--) // Loop for spaces
{
Console.Write(" "); // Displaying spaces
}
// Loop to display numbers in the pattern
for (j = 1; j <= i; j++)
{
Console.Write("{0} ", i); // Displaying the repeated number in the same row
}
Console.Write("\n"); // Moving to the next line for the next row
spc--; // Decrementing space for the next row
}
}
}
Sample Output:
Display the pattern like pyramid with repeating a number in same row: ----------------------------------------------------------------------- Input number of rows : 8 1 2 2 3 3 3 4 4 4 4 5 5 5 5 5 6 6 6 6 6 6 7 7 7 7 7 7 7 8 8 8 8 8 8 8 8
Flowchart:
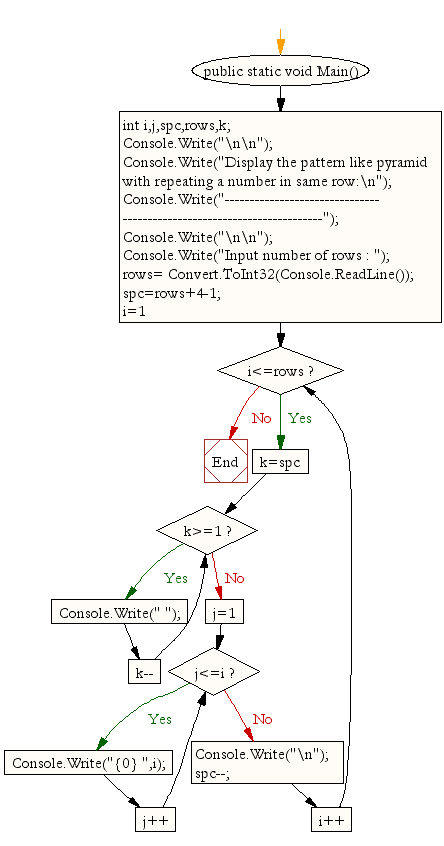
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to display the n terms of even natural number and their sum.
Next: Write a program in C# Sharp to find the sum of the series [ 1-X^2/2!+X^4/4!- .........].
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/for-loop/csharp-for-loop-exercise-17.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics