C#: Calculate n terms of even natural number and their sum
Write a program in C# Sharp to display the n terms of even natural number and their sum.
Visual Presentation:
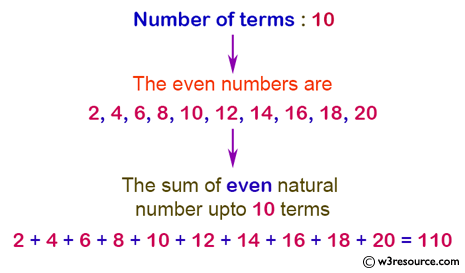
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise16 // Declaration of the Exercise16 class
{
public static void Main() // Main method, entry point of the program
{
int i, n, sum = 0; // Declaration of variables 'i' for iteration, 'n' for the number of terms, 'sum' to store the sum
Console.Write("\n\n"); // Displaying new lines
Console.Write("Calculate n terms of even natural number and their sum:\n"); // Displaying the purpose of the program
Console.Write("---------------------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input number of terms : "); // Prompting the user to input the number of terms
n = Convert.ToInt32(Console.ReadLine()); // Reading the number of terms entered by the user
Console.Write("\nThe even numbers are :"); // Displaying a message for even numbers
for (i = 1; i <= n; i++) // Loop to calculate even numbers and their sum
{
Console.Write("{0} ", 2 * i); // Displaying the even number in the sequence
sum += 2 * i; // Adding the even number to the sum
}
Console.Write("\nThe Sum of even Natural Number up to {0} terms : {1} \n", n, sum); // Displaying the sum of even natural numbers
}
}
Sample Output:
Calculate n terms of even natural number and their sum: --------------------------------------------------------- Input number of terms : 10 The even numbers are :2 4 6 8 10 12 14 16 18 20 The Sum of even Natural Number upto 10 terms : 110
Flowchart:
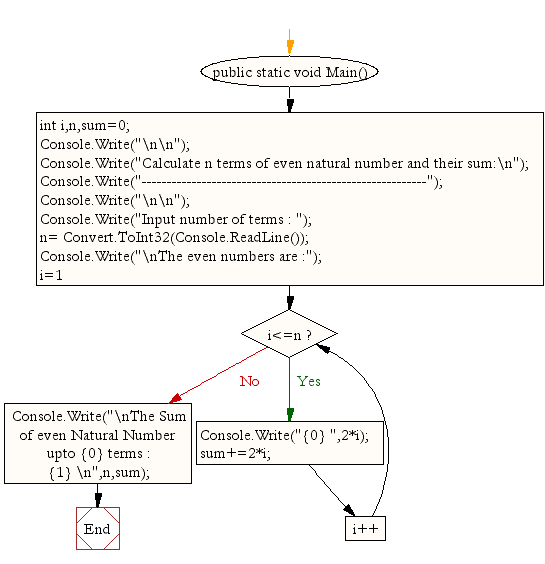
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to calculate the factorial of a given number.
Next: Write a program in C# Sharp to make such a pattern like a pyramid with number which will repeat the number in the same row
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.