C#: Calculate the factorial of a given number
C# Sharp For Loop: Exercise-15 with Solution
Write a C# Sharp program to calculate the factorial of a given number.
Visual Presentation:
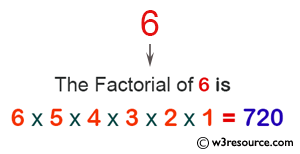
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise15 // Declaration of the Exercise15 class
{
public static void Main() // Main method, entry point of the program
{
int i, f = 1, num; // Declaration of variables 'i' for iteration, 'f' to store factorial, 'num' to store the input number
Console.Write("\n\n"); // Displaying new lines
Console.Write("Calculate the factorial of a given number:\n"); // Displaying the purpose of the program
Console.Write("--------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input the number : "); // Prompting the user to input a number
num = Convert.ToInt32(Console.ReadLine()); // Reading the number entered by the user
for (i = 1; i <= num; i++) // Loop to calculate the factorial of the input number
{
f = f * i; // Multiplying 'f' by 'i' in each iteration to calculate factorial
}
Console.Write("The Factorial of {0} is: {1}\n", num, f); // Displaying the factorial of the entered number
}
}
Sample Output:
Calculate the factorial of a given number: -------------------------------------------- Input the number : 6 The Factorial of 6 is: 720
Flowchart:
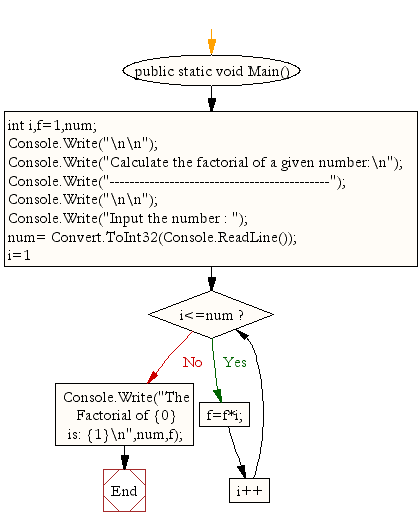
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to make such a pattern like a pyramid with an asterisk.
Next: Write a program in C# Sharp to display the n terms of even natural number and their sum.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/for-loop/csharp-for-loop-exercise-15.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics