Java: Find the length of last word of a specified string
Java Basic: Exercise-181 with Solution
Length of Last Word
Write a Java program to find the length of the last word in a given string. The string contains upper/lower-case alphabets and empty space characters like ' '.
Visual Presentation:
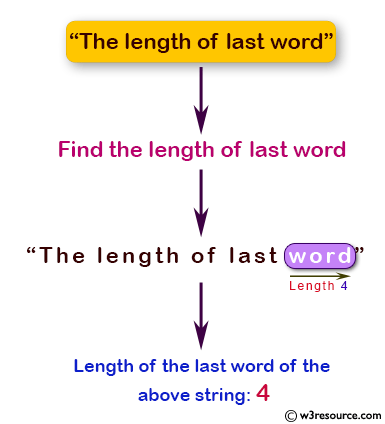
Sample Solution:
Java Code:
// Importing necessary Java utilities
import java.util.*;
// Main class Solution
public class Solution {
// Main method
public static void main(String[] args) {
// Initializing a string
String str1 = "The length of last word";
// Printing the original string
System.out.println("Original String: " + str1);
// Printing the length of the last word of the string
System.out.println("Length of the last word of the above string: " + length_Of_last_word(str1));
}
// Method to calculate the length of the last word in a string
public static int length_Of_last_word(String str1) {
int length_word = 0; // Initializing the variable to store the length of the last word
String[] words = str1.split(" "); // Splitting the string into words based on spaces
// Checking if words exist in the array after splitting
if (words.length > 0) {
// Assigning the length of the last word to the variable
length_word = words[words.length - 1].length();
} else {
length_word = 0; // If no words are present, setting the length to 0
}
return length_word; // Returning the length of the last word
}
}
Sample Output:
Original String: The length of last word Length of the last word of the above string: 4
Flowchart:
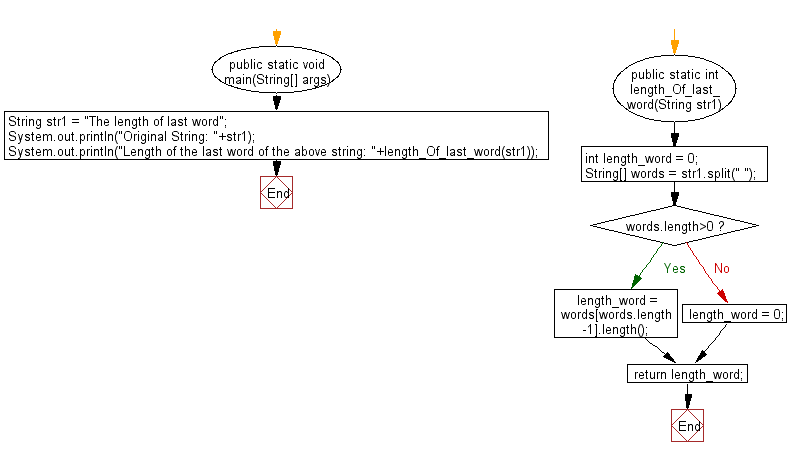
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to swap every two adjacent nodes of a given linked list.
Next: Write a Java program to check if two binary trees are identical or not. Assume that two binary trees have the same structure and every identical position has the same value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/basic/java-basic-exercise-181.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics