JavaFX Month days calculator
JavaFx User Interface Components: Exercise-8 with Solution
Write a JavaFX application that uses a ComboBox to choose a month (e.g., January, February). When a month is selected, display the number of days in that month.
Sample Solution:
JavaFx Code:
//Main.java
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.scene.Scene;
import javafx.scene.control.ComboBox;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
import java.util.HashMap;
import java.util.Map;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Month Days App");
// Create a label to display the number of days.
Label daysLabel = new Label("Number of Days: ");
// Create a ComboBox with a list of months.
ComboBox monthComboBox = new ComboBox<>(FXCollections.observableArrayList(
"January", "February", "March", "April", "May", "June",
"July", "August", "September", "October", "November", "December"
));
// Create a map to store the number of days for each month.
Map monthDaysMap = new HashMap<>();
monthDaysMap.put("January", 31);
monthDaysMap.put("February", 28);
monthDaysMap.put("March", 31);
monthDaysMap.put("April", 30);
monthDaysMap.put("May", 31);
monthDaysMap.put("June", 30);
monthDaysMap.put("July", 31);
monthDaysMap.put("August", 31);
monthDaysMap.put("September", 30);
monthDaysMap.put("October", 31);
monthDaysMap.put("November", 30);
monthDaysMap.put("December", 31);
// Handle the action when a month is selected from the ComboBox.
monthComboBox.setOnAction(event -> {
String selectedMonth = monthComboBox.getValue();
int days = monthDaysMap.get(selectedMonth);
daysLabel.setText("Number of Days in " + selectedMonth + ": " + days);
});
// Create a layout (VBox) to arrange the ComboBox and label.
VBox root = new VBox(10);
root.getChildren().addAll(monthComboBox, daysLabel);
// Create the scene and set it in the stage.
Scene scene = new Scene(root, 300, 200);
primaryStage.setScene(scene);
// Set the title of the window.
primaryStage.show();
}
}
In the above JavaFX application, we use a ComboBox to select a month. A map is used to store the number of days in each month. When a month is selected from the ComboBox, it retrieves the number of days from the map and displays it in a label. The elements are organized using a 'VBox'.
Sample Output:
Flowchart:
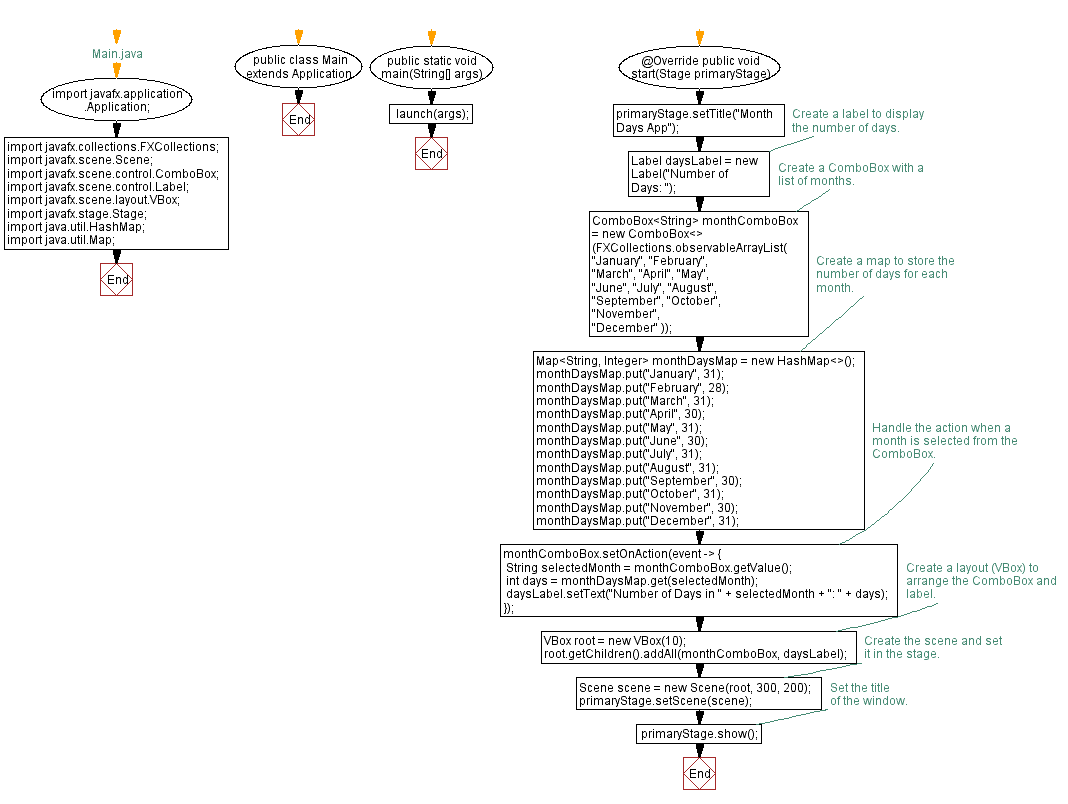
Java Code Editor:
Previous: JavaFX Color choice application.
Next: JavaFX Country selection application.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics