Java: Manage a music library of songs
Java OOP: Exercise-15 with Solution
Write a Java program to create a class called "MusicLibrary" with a collection of songs and methods to add and remove songs, and to play a random song.
Sample Solution:
Java Code:
// MusicLibrary.java
// Import the ArrayList class from the Java utility package
import java.util.ArrayList;
// Import the Random class from the Java utility package
import java.util.Random;
// Define the MusicLibrary class
public class MusicLibrary {
// Declare a private field to store a list of Song objects
private ArrayList<Song> songs;
// Constructor to initialize the songs list
public MusicLibrary() {
// Create a new ArrayList to hold Song objects
songs = new ArrayList<Song>();
}
// Method to add a song to the library
public void addSong(Song song) {
// Add the provided song to the songs list
songs.add(song);
}
// Method to remove a song from the library
public void removeSong(Song song) {
// Remove the provided song from the songs list
songs.remove(song);
}
// Method to get the list of all songs in the library
public ArrayList<Song> getSongs() {
// Return the list of songs
return songs;
}
// Method to play a random song from the library
public void playRandomSong() {
// Get the number of songs in the library
int size = songs.size();
// Check if the library is empty
if (size == 0) {
// Print a message if there are no songs to play
System.out.println("No songs in the library.");
return; // Exit the method
}
// Create a new Random object to generate a random number
Random rand = new Random();
// Generate a random index within the range of the songs list
int index = rand.nextInt(size);
// Print the title and artist of the randomly selected song
System.out.println("Now playing: " + songs.get(index).getTitle() + " by " + songs.get(index).getArtist());
}
}
The above “MusicLibrary” class represents a library of songs. It uses an ArrayList to store the songs, and provides methods to add and remove songs from the library. It also has a method to get a list of all the songs in the library, and a method to play a random song from the library.
// Song.java
// Define the Song class
public class Song {
// Private fields to store the title and artist of the song
private String title;
private String artist;
// Constructor to initialize the title and artist fields
public Song(String title, String artist) {
this.title = title; // Set the title field to the provided title
this.artist = artist; // Set the artist field to the provided artist
}
// Getter method to retrieve the title of the song
public String getTitle() {
return title; // Return the value of the title field
}
// Setter method to update the title of the song
public void setTitle(String title) {
this.title = title; // Set the title field to the provided title
}
// Getter method to retrieve the artist of the song
public String getArtist() {
return artist; // Return the value of the artist field
}
// Setter method to update the artist of the song
public void setArtist(String artist) {
this.artist = artist; // Set the artist field to the provided artist
}
}
The “Song” class represents a song in a music library. It has two private instance variables, the title and the artist, and a constructor that takes these two variables as parameters. The class also provides getters and setters for the title and artist variables, allowing the client code to access and modify the song information.
// Main.java
// Import the ArrayList class from the Java utility package
import java.util.ArrayList;
// Define the Main class
public class Main {
// The main method - the entry point of the Java application
public static void main(String[] args) {
// Create a new instance of the MusicLibrary class
MusicLibrary library = new MusicLibrary();
// Add songs to the music library
library.addSong(new Song("Midnight Train to Georgia", "Gladys Knight & the Pips"));
library.addSong(new Song("Stairway to Heaven", "Led Zeppelin"));
library.addSong(new Song("Imagine", "John Lennon"));
library.addSong(new Song("All by Myself", "Eric Carmen"));
library.addSong(new Song("What'd I Say", "Ray Charles"));
library.addSong(new Song("Walking in Memphis", "Marc Cohn"));
library.addSong(new Song("In the Air Tonight", "Phil Collins"));
// Print all songs in the music library
System.out.println("All songs:");
// Iterate through each song in the library and print its title and artist
for (Song song : library.getSongs()) {
System.out.println(song.getTitle() + " by " + song.getArtist());
}
// Print a message indicating a random song will be played
System.out.println("\n**Playing Random Song**");
// Play and print a random song from the library three times
library.playRandomSong();
System.out.println();
library.playRandomSong();
System.out.println();
library.playRandomSong();
}
}
The Main class creates an instance of MusicLibrary and adds several Song objects to it. It then retrieves and prints out the list of all songs in the library. Finally, it calls the playRandomSong() method of the MusicLibrary object three times to play a random song each time, printing out the details of the played song.
Sample Output:
All songs: Midnight Train to Georgia by Gladys Knight & the Pips Stairway to Heaven by Led Zeppelin Imagine by John Lennon All by Myself by Eric Carmen What'd I Say by Ray Charles Walking in Memphis by Marc Cohn In the Air Tonight by Phil Collins **Playing Random Song** Now playing: Imagine by John Lennon Now playing: Midnight Train to Georgia by Gladys Knight & the Pips Now playing: What'd I Say by Ray Charles
Flowchart:
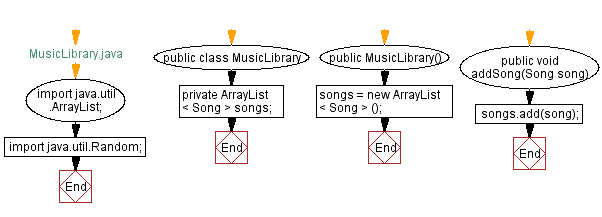
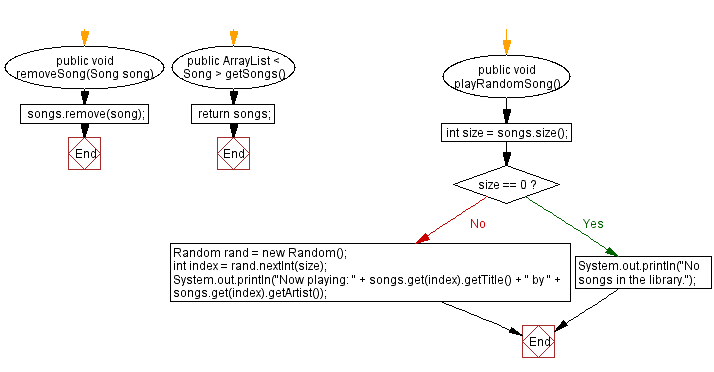
Java Code Editor:
Improve this sample solution and post your code through Disqus.
Java OOP Previous: School Management System.
Java OOP Next: Calculate the area and perimeter of shapes.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/oop/java-oop-exercise-15.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics