Java Recursive Method: Calculate the factorial of a number
Java Recursive: Exercise-1 with Solution
Write a Java recursive method to calculate the factorial of a given positive integer.
Sample Solution:
Java Code:
public class FactorialCalculator {
public static int calculateFactorial(int n) {
// Base case: factorial of 0 is 1
if (n == 0) {
return 1;
}
// Recursive case: multiply n with factorial of (n-1)
return n * calculateFactorial(n - 1);
}
public static void main(String[] args) {
int number = 7;
int factorial = calculateFactorial(number);
System.out.println("Factorial of " + number + " is: " + factorial);
number = 12;
factorial = calculateFactorial(number);
System.out.println("\nFactorial of " + number + " is: " + factorial);
}
}
Sample Output:
Factorial of 7 is: 5040 Factorial of 12 is: 479001600
Explanation:
In the above exercises -
First, we define a class "FactorialCalculator" that includes a recursive method calculateFactorial() to calculate the factorial of a given positive integer n.
The calculateFactorial() method follows the recursive factorial definition. It has two cases:
- Base case: If n is 0, it returns 1. This is the termination condition for recursion.
- Recursive case: For any positive n, it multiplies n with the factorial of n-1. This step is repeated recursively until n reaches 0.
In the main() method, we demonstrate the calculateFactorial() method by calculating the factorial of 7 and 12.
Flowchart:
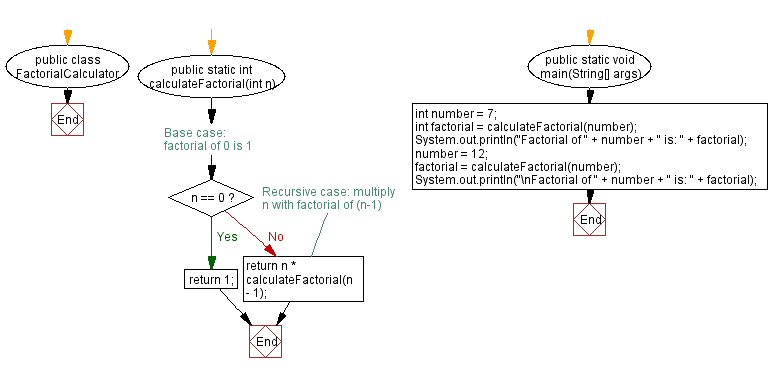
Java Code Editor:
Java Recursive Previous: Java Recursive Exercises Home.
Java Recursive Next: Calculate the sum of numbers from 1 to n.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics