Java Recursive Method: Calculate the sum of numbers from 1 to n
Java Recursive: Exercise-2 with Solution
Write a Java recursive method to calculate the sum of all numbers from 1 to n.
Sample Solution:
Java Code:
public class SumCalculator {
public static int calculateSum(int n) {
// Base case: sum of 0 is 0
if (n == 0) {
return 0;
}
// Recursive case: add n with the sum of (n-1)
return n + calculateSum(n - 1);
}
public static void main(String[] args) {
int number = 7;
int sum = calculateSum(number);
System.out.println("Sum of numbers from 1 to " + number + " is: " + sum);
}
}
Sample Output:
Sum of numbers from 1 to 7 is: 28
Explanation:
In the above exercises -
The calculateSum() method follows the recursive definition of the sum. It has two cases:
- Base case: If the input n is 0, it returns 0. This is the termination condition for recursion.
- Recursive case: For any positive n, it adds n with the sum of the numbers from 1 to n-1. This step is repeated recursively until n reaches 0
In the main() method, we demonstrate the calculateSum() method by calculating the sum of numbers from 1 to 7 and printing the result.
Flowchart:
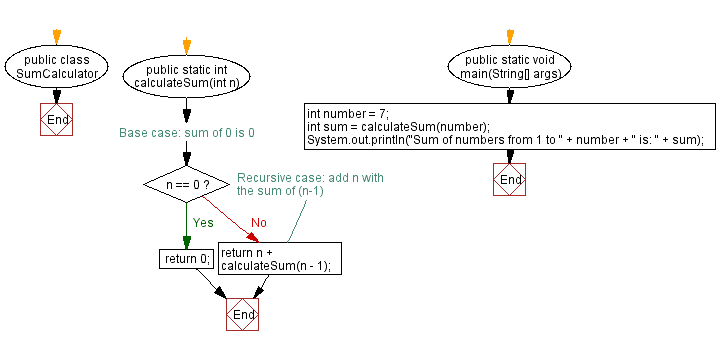
Java Code Editor:
Java Recursive Previous: Calculate the factorial of a number.
Java Recursive Next: Calculate the nth Fibonacci number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics