JavaScript: Run a function in a separate thread by using a Web Worker, allowing long running functions to not block the UI
JavaScript: Exercise-151 with Solution
Run Function in Web Worker
Write a JavaScript program to run a function in a separate thread using a Web Worker. This allows long running functions to not block the UI.
Note: Create a new Worker using a Blob object URL, the contents of which should be the stringified version of the supplied function. Immediately post the return value of calling the function back. Return a promise, listening for onmessage and onerror events and resolving the data posted back from the worker, or throwing an error.
Since the function is running in a different context, closures are not supported. The function supplied to `runAsync` gets stringified, so everything becomes literal. All variables and functions must be defined inside.
- Create a new Worker() using a Blob object URL, the contents of which should be the stringified version of the supplied function.
- Immediately post the return value of calling the function back.
- Return a new Promise(), listening for onmessage and onerror events and resolving the data posted back from the worker, or throwing an error.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the runAsync function
const runAsync = fn => {
// Create a new worker with the given function
const worker = new Worker(
URL.createObjectURL(new Blob([`postMessage((${fn})());`]), {
type: 'application/javascript; charset=utf-8'
})
);
// Return a promise that resolves with the result of the function
return new Promise((res, rej) => {
// Listen for messages from the worker
worker.onmessage = ({ data }) => {
res(data), worker.terminate(); // Resolve the promise with the data and terminate the worker
};
// Listen for errors from the worker
worker.onerror = err => {
rej(err), worker.terminate(); // Reject the promise with the error and terminate the worker
};
});
};
// Define a long-running function
const longRunningFunction = () => {
let result = 0;
for (let i = 0; i < 1000; i++) {
for (let j = 0; j < 700; j++) {
for (let k = 0; k < 300; k++) {
result = result + i + j + k;
}
}
}
return result;
};
// Example usage of runAsync with different functions
runAsync(longRunningFunction).then(console.log);
runAsync(() => 10 ** 3).then(console.log);
let outsideVariable = 50;
runAsync(() => typeof outsideVariable).then(console.log);
Sample Output:
1000 undefined
Flowchart:
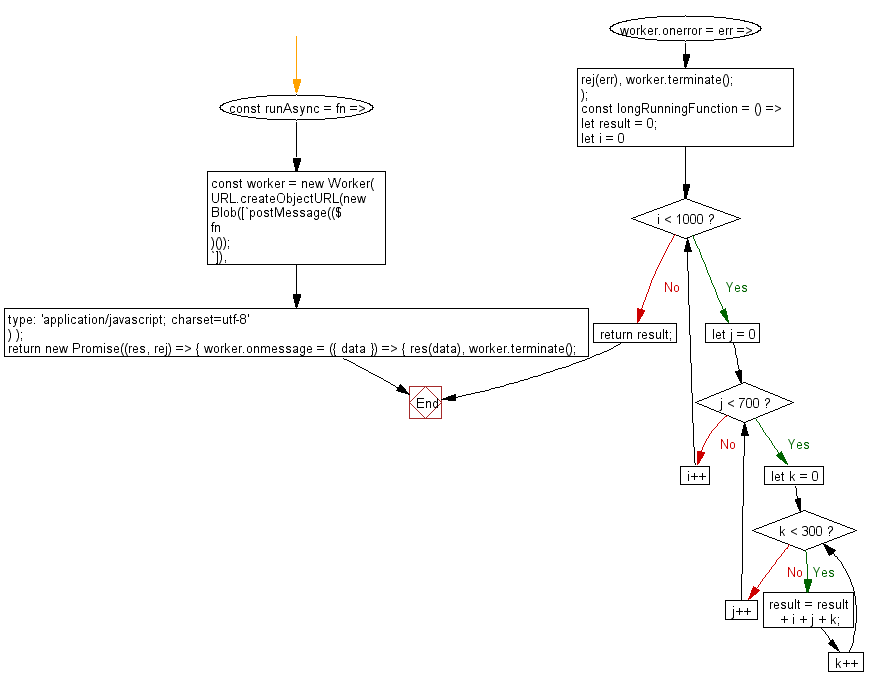
Live Demo:
See the Pen javascript-basic-exercise-151-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that offloads a CPU-intensive calculation (e.g., prime number generation) to a Web Worker and returns the result to the main thread.
- Write a JavaScript program that creates a Web Worker to process large JSON data, sending periodic progress updates back to the UI.
- Write a JavaScript program that utilizes transferable objects (e.g., ArrayBuffer) with a Web Worker to optimize data processing speed.
- Write a JavaScript program that spawns a Web Worker to perform a recursive computation (like calculating factorial for a large number) without blocking the UI.
- Write a JavaScript program that demonstrates error handling in a Web Worker by intentionally triggering an error and catching it in the main thread.
Go to:
PREV : Run Promises in Series.
NEXT : Round Number to Digits.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.