JavaScript: Perform left-to-right function composition
JavaScript fundamental (ES6 Syntax): Exercise-165 with Solution
Left-to-Right Function Composition
Write a JavaScript program to perform left-to-right function composition.
- Use Array.prototype.reduce() with the spread operator (...) to perform left-to-right function composition.
- The first (leftmost) function can accept one or more arguments; the remaining functions must be unary.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'pipeFunctions' that takes any number of functions as arguments and returns a new function
const pipeFunctions = (...fns) => fns.reduce((f, g) => (...args) => g(f(...args)));
// Define a function 'add5' that adds 5 to its argument
const add5 = x => x + 5;
// Define a function 'multiply' that multiplies its two arguments
const multiply = (x, y) => x * y;
// Create a new function 'multiplyAndAdd5' by piping the 'multiply' function followed by the 'add5' function
const multiplyAndAdd5 = pipeFunctions(multiply, add5);
// Call 'multiplyAndAdd5' with arguments 5 and 2, then log the result to the console
console.log(multiplyAndAdd5(5, 2));
// Call 'multiplyAndAdd5' with arguments 16 and 2, then log the result to the console
console.log(multiplyAndAdd5(16, 2));
Sample Output:
15 37
Pictorial Presentation:
Flowchart:
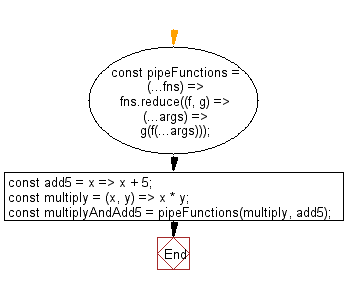
Live Demo:
See the Pen javascript-basic-exercise-165-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that composes multiple functions from left to right and returns a new function.
- Write a JavaScript function that implements a pipeline where each function’s output is passed as input to the next.
- Write a JavaScript program that uses Array.reduce() to perform left-to-right function composition.
- Write a JavaScript function that composes a list of functions and applies them sequentially to an initial value.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program that will return the singular or plural form of the word based on the input number.
Next: Write a JavaScript program to perform left-to-right function composition for asynchronous functions.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.