JavaScript: Perform left-to-right function composition for asynchronous functions
JavaScript fundamental (ES6 Syntax): Exercise-166 with Solution
Async Left-to-Right Composition
Write a JavaScript program to perform left-to-right function composition for asynchronous functions.
- Use Array.prototype.reduce() and the spread operator (...) to perform function composition using Promise.prototype.then().
- The functions can return a combination of normal values, Promises or be async, returning through await.
- All functions must accept a single argument.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'pipeAsyncFunctions' that takes any number of asynchronous functions as arguments and returns a new asynchronous function
const pipeAsyncFunctions = (...fns) => arg => fns.reduce((p, f) => p.then(f), Promise.resolve(arg));
// Define a chain of asynchronous functions to be composed together
const sum = pipeAsyncFunctions(
x => x + 1, // Adds 1 to the input
x => new Promise(resolve => setTimeout(() => resolve(x + 2), 1000)), // Adds 2 to the input after a delay of 1 second
x => x + 3, // Adds 3 to the input
async x => (await x) + 4 // Adds 4 to the input asynchronously
);
// Call the composed asynchronous function with an initial value of 5 and log the result to the console
(async () => {
console.log(await sum(5)); // 15 (after one second)
})();
Sample Output:
15
Flowchart:
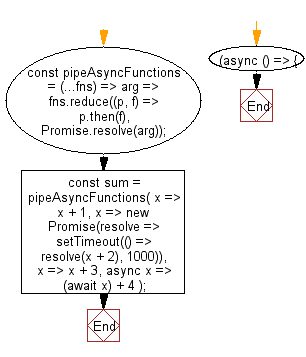
Live Demo:
See the Pen javascript-fundamental-exercise-166 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that composes asynchronous functions in left-to-right order using async/await.
- Write a JavaScript function that chains promises in a left-to-right manner to process an initial value.
- Write a JavaScript program that builds a pipeline of asynchronous functions, ensuring each awaits the previous.
- Write a JavaScript function that accepts an array of async functions and returns a new function that processes input sequentially.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to perform left-to-right function composition.
Next: Write a JavaScript program to calculate how many numbers in the given array are less or equal to the given value using the percentile formula.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.